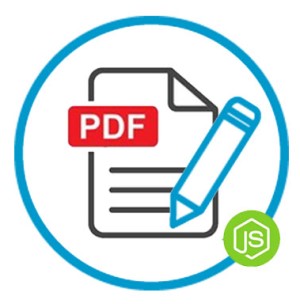
As a Node.js developer, you can easily annotate any of your PDF documents programmatically on the cloud. You can add images, comments, notes, or other types of external remarks to the document as annotations. In this article, you will learn how to annotate PDF documents using a REST API in Node.js.
The following topics shall be covered in this article:
- Document Annotation REST API and Node.js SDK
- Annotate PDF Documents using a REST API in Node.js
- Add Image Annotations using Node.js
Document Annotation REST API and Node.js SDK
For annotating PDF documents, I will be using the Node.js SDK of GroupDocs.Annotation Cloud API. It allows you to programmatically build document annotation tools online. You can add annotations, watermark overlays, text replacements, redactions, and text markups to the supported document formats. It also provides .NET, Java, PHP, Ruby, and Python SDKs as its document annotation family members for the Cloud API.
You can install GroupDocs.Annotation Cloud to your Node.js application using the following command in the console:
npm install groupdocs-annotation-cloud
Please get your Client ID and Client Secret from the dashboard before you start following the steps and available code examples. Once you have your ID and secret, add in the code as shown below:
Annotate PDF Documents using a REST API in Node.js
You can annotate your PDF documents on the cloud by following the simple steps given below:
- Upload the PDF file to the Cloud
- Annotate PDF Document using Node.js
- Download the annotated file
Upload the Document
Firstly, upload the PDF file to the Cloud using the following code sample:
As a result, the uploaded PDF file will be available in the files section of your dashboard on the cloud.
Annotate PDF Document using Node.js
You can add multiple annotations to the PDF document programmatically by following the steps mentioned below:
- Create an instance of the AnnotateApi
- Create the first instance of the AnnotationInfo
- Set annotation properties for the first instance e.g. position, type, text, etc.
- Create second instance of the AnnotationInfo
- Set annotation properties for the second instance e.g. position, type, text, etc.
- Create third instance of the AnnotationInfo
- Set annotation properties for the third instance e.g. position, type, text, etc.
- Create fourth instance of the AnnotationInfo
- Set annotation properties for the fourth instance e.g. position, type, text, etc.
- Create a FileInfo instance and set the input file path
- Create an instance of the AnnotateOptions
- Assign the FileInfo and defined annotation instances to the AnnotateOptions
- Set the output file path
- Create a request by calling the AnnotateRequest method with AnnotateOptions
- Get results by calling the AnnotateApi.annotate() method with AnnotateRequest
The following code sample shows how to annotate a PDF document with multiple annotations using a REST API in Node.js.
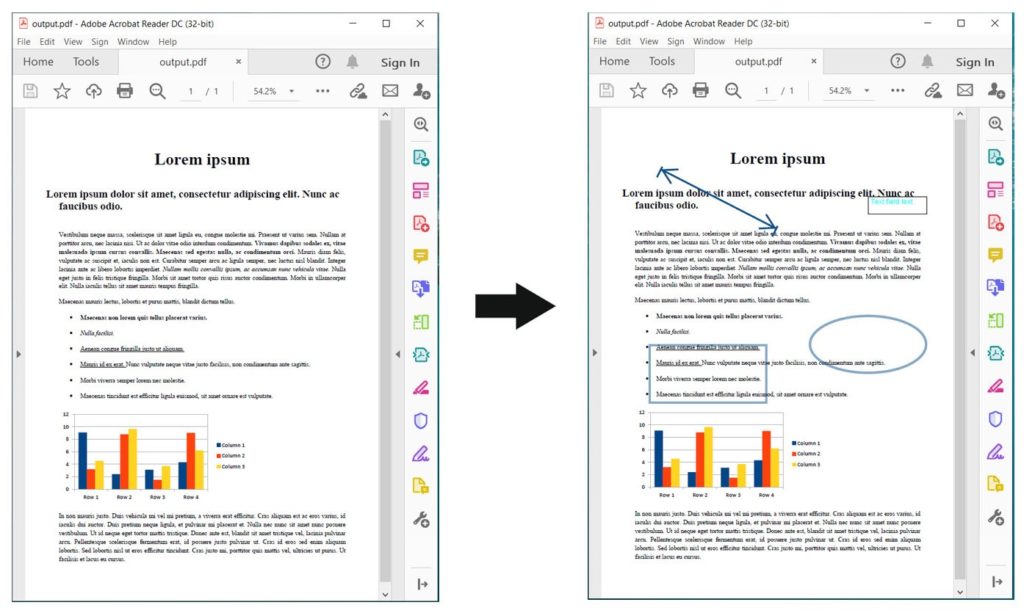
Annotate PDF Documents using a REST API in Node.js
You can read more about supported annotation types under adding annotations section in the documentation.
Download the Annotated File
The above code sample will save the annotated PDF file on the cloud. You can download it using the following code sample:
Add Image Annotations using Node.js
You can add image annotations in your PDF documents programmatically by following the steps given below:
- Create an instance of the AnnotateApi
- Create an instance of the AnnotationInfo
- Define a rectangle and set its position, height, and width
- Set annotation properties e.g. position, text, height, width, etc.
- Set the annotation type as Image
- Create a FileInfo instance and set the input file path
- Create an instance of the AnnotateOptions
- Assign the FileInfo and annotation to the AnnotateOptions
- Set the output file path
- Create a request by calling the AnnotateRequest method with AnnotateOptions
- Get results by calling the AnnotateApi.annotate() method with AnnotateRequest
The following code sample shows how to add image annotations in the PDF document using a REST API in Node.js. Please follow the steps mentioned earlier to upload and download a file.
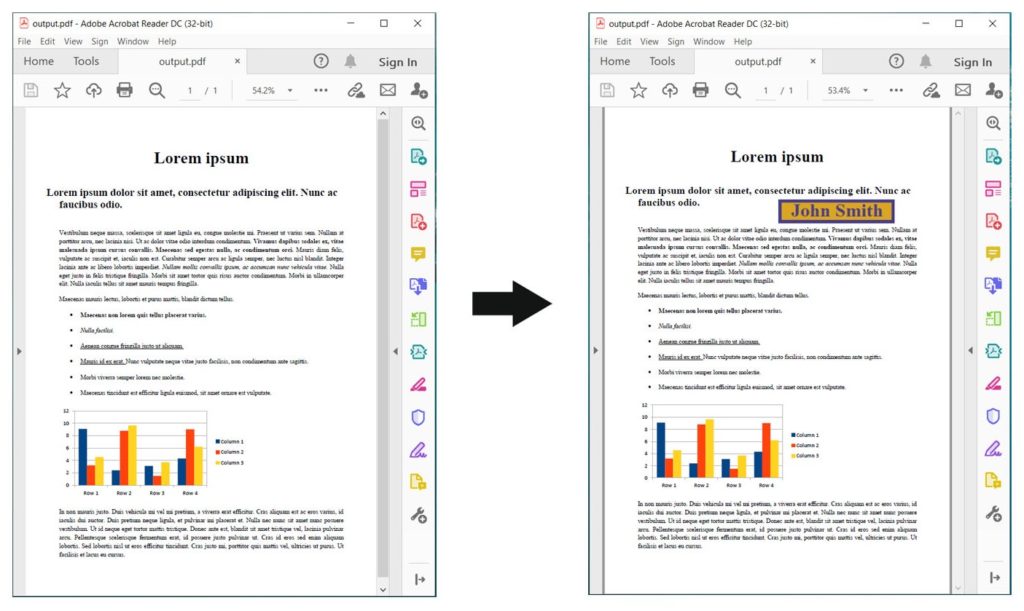
Add Image Annotations using Node.js
Try Online
Please try the following free online PDF annotation tool, which is developed using the above API. https://products.groupdocs.app/annotation/pdf
Conclusion
In this article, you have learned how to add multiple annotations to PDF documents on the cloud. You have also learned how to add image annotations to PDF documents programmatically. Moreover, you have learned how to programmatically upload a PDF file on the cloud and then download the annotated file from the cloud. You can learn more about GroupDocs.Annotation Cloud API using the documentation. We also provide an API Reference section that lets you visualize and interact with our APIs directly through the browser. In case of any ambiguity, please feel free to contact us on the forum.