
You can annotate any PDF document programmatically on the cloud using Python. It can be any additional information about an existing piece of data in the form of images, comments, notes, or other types of external remarks in the document. In this article, you will learn how to annotate PDF documents using a REST API in Python.
The following topics shall be covered in this article:
- Document Annotation REST API and Python SDK
- Annotate PDF Documents using a REST API in Python
- Add TextField Annotations using Python
- Add Image Annotations using Python
- Annotate with Link Annotations using Python
Document Annotation REST API and Python SDK
For annotating PDF documents, I will be using the Python SDK of GroupDocs.Annotation Cloud API. It allows to programmatically build document annotation tools online. You can add annotations, watermark overlays, text replacements, redactions, and text markups to the business documents of all popular formats. It also provides .NET, Java, PHP, Ruby, and Node.js SDKs as its document annotation family members for the Cloud API.
You can install GroupDocs.Annotation Cloud to your Python project using the following command in the console:
pip install groupdocs_annotation_cloud
Please get your Client ID and Client Secret from the dashboard before you start following the steps and available code examples. Once you have your ID and secret, add in the code as shown below:
Annotate PDF Documents using a REST API in Python
You can add annotations to your PDF Documents by following the simple steps given below:
- Upload the PDF file to the Cloud
- Annotate PDF Documents using Python
- Download the annotated file
Upload the Document
Firstly, upload the PDF file to the Cloud using the following code sample:
As a result, the uploaded PDF file will be available in the files section of your dashboard on the cloud.
Annotate PDF Documents using Python
Please follow the steps mentioned below to add multiple annotations to the PDF document programmatically.
- Create an instance of AnnotateApi
- Create the first instance of AnnotationInfo
- Set annotation properties for the first instance e.g. position, type, text, etc.
- Create AnnotationInfo second instance
- Set annotation properties for the second instance e.g. position, type, text, etc.
- Create AnnotationInfo third instance
- Set annotation properties for the third instance e.g. position, type, text, etc.
- Create a FileInfo instance and set the input file path
- Create an instance of AnnotateOptions and set file info to AnnotateOptions
- Assign first, second, and third annotations to AnnotateOptions
- Create a request by calling the AnnotateRequest method
- Get results by calling the AnnotateApi.annotate() method
The following code sample shows how to annotate a PDF document and add multiple annotations using a REST API.
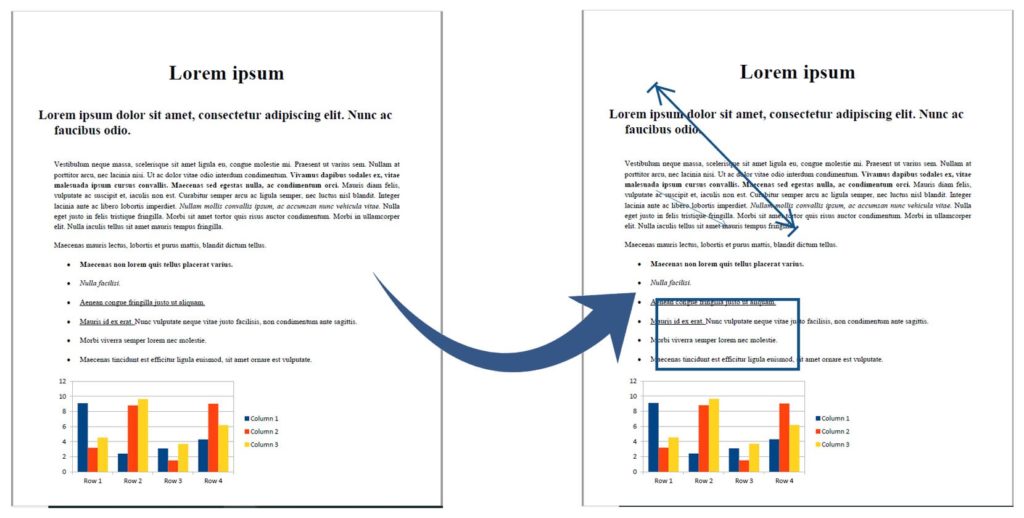
Add Multiple Annotations to PDF Document using a REST API in Python
You can read more about supported annotation types under adding annotations section in the documentation.
Download the Annotated File
The above code sample will save the annotated PDF file on the cloud. You can download it using the following code sample:
Add TextField Annotations using Python
You can add Text field annotations in the PDF documents programmatically by following the steps given below:
- Create an instance of AnnotateApi
- Create an instance of AnnotationInfo
- Define annotation position
- Define Rectangle position, height, and width
- Set various Annotation properties e.g. text, height, width, etc.
- Set annotation type as TextField
- Create a FileInfo instance and set the input file path
- Create an instance of AnnotateOptions and set file info to AnnotateOptions
- Assign annotation to AnnotateOptions
- Create a request by calling the AnnotateRequest method
- Get results by calling the AnnotateApi.annotate() method
The following code sample shows how to add text field annotations in the PDF document using a REST API. Please follow the steps mentioned earlier to upload and download a file.
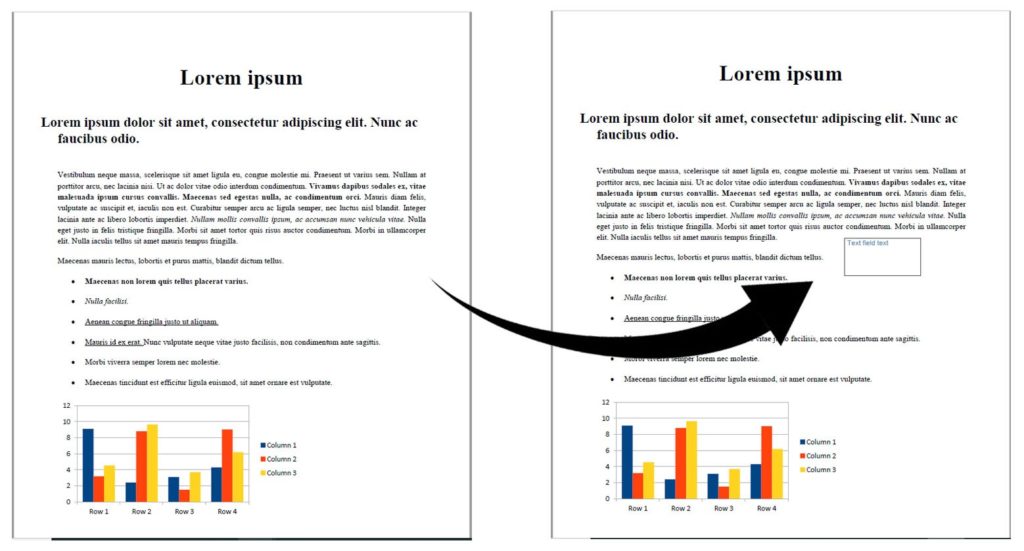
Add Text Field Annotations using Python
Add Image Annotations using Python
You can add image annotations in your PDF documents programmatically by following the steps given below:
- Create an instance of AnnotateApi
- Create an instance of AnnotationInfo
- Define Rectangle and set its position, height, and width
- Set various Annotation properties e.g. position, text, height, width, etc.
- Set annotation type as Image
- Create a FileInfo instance and set the input file path
- Create an instance of AnnotateOptions and set file info to AnnotateOptions
- Assign annotation to AnnotateOptions
- Create a request by calling the AnnotateRequest method
- Get results by calling the AnnotateApi.annotate() method
The following code sample shows how to add image annotations in the PDF document using a REST API. Please follow the steps mentioned earlier to upload and download a file.
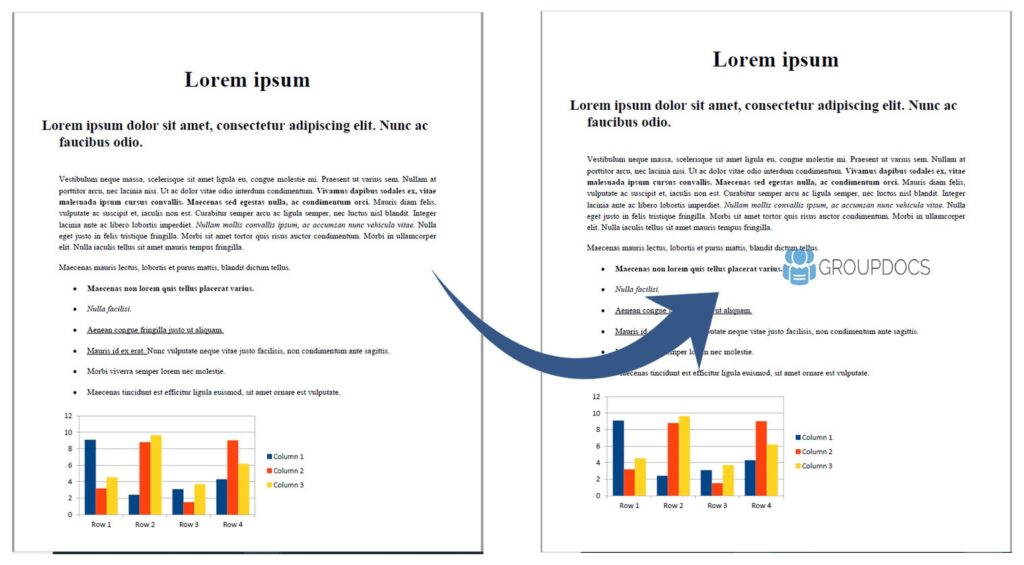
Add Image Annotations using Python
Annotate with Link Annotations using Python
You can add hyperlink annotations in the PDF documents programmatically by following the steps given below:
- Create an instance of AnnotateApi
- Create an instance of AnnotationInfo
- Define annotation points and set position for each point
- Set various Annotation properties e.g. text, height, width, etc.
- Set annotation type as Link
- Create a FileInfo instance and set the input file path
- Create an instance of AnnotateOptions and set file info to AnnotateOptions
- Assign annotation to AnnotateOptions
- Create a request by calling the AnnotateRequest method
- Get results by calling the AnnotateApi.annotate() method
The following code sample shows how to add hyperlink annotations in the PDF document using a REST API. Please follow the steps mentioned earlier to upload and download a file.
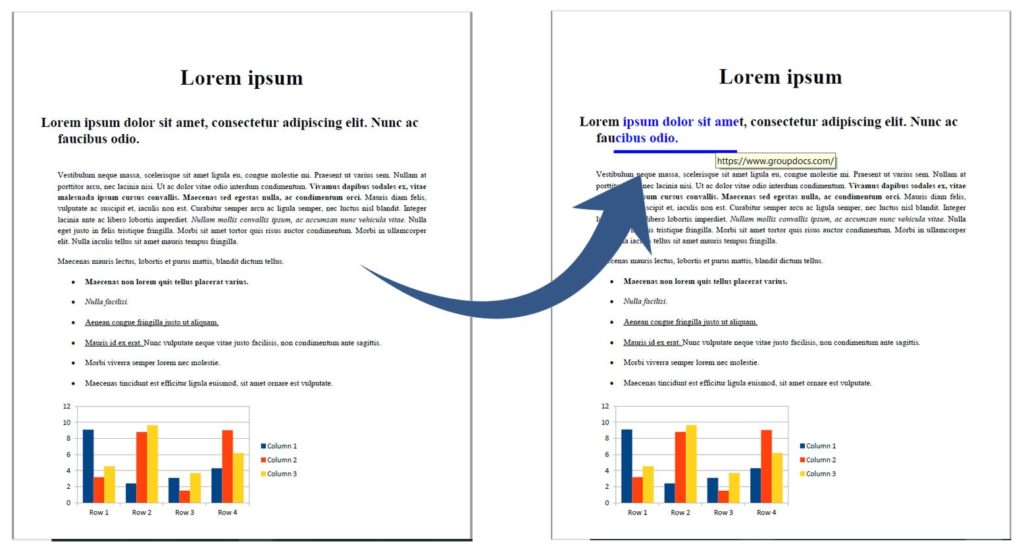
Annotate with Link Annotations using Python
Try Online
Please try the following free online PDF annotation tool, which is developed using the above API. https://products.groupdocs.app/annotation/pdf
Conclusion
In this article, you have learned how to add various types of annotations to PDF documentson the cloud. Furthermore, you have learned how to programmatically upload the PDF file on the cloud and then download the annotated file from the cloud. You can learn more about GroupDocs.Annotation Cloud API using the documentation. We also provide an API Reference section that lets you visualize and interact with our APIs directly through the browser. In case of any ambiguity, please feel free to contact us on the forum.