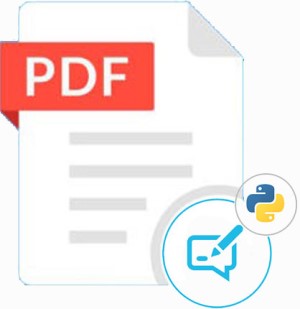
Annotations provide additional information in the document in the form of comments, popups, and various other graphical objects. In some cases, we may need to remove annotations from annotated PDF documents. In this article, we will learn how to remove or extract annotations from PDF documents using a REST API in Python.
The following topics shall be covered in this article:
- REST API and Python SDK to Remove Annotations
- Remove Annotations from PDF Files using a REST API in Python
- Extract Annotations from PDF Documents in Python
REST API and Python SDK to Remove Annotations
For extracting or removing the annotations from PDF documents, we will be using the Python SDK of GroupDocs.Annotation Cloud API. It allows adding annotations, watermark overlays, text replacements & markups, and sticky notes to the supported documents formats. Please install it using the following command in the console:
pip install groupdocs_annotation_cloud
Please get your Client ID and Secret from the dashboard before following the mentioned steps. Once you have your ID and secret, add in the code as shown below:
Remove Annotations from PDF Files using a REST API in Python
We can delete all the annotations from PDF files by following the simple steps mentioned below:
- Upload the PDF file to the Cloud
- Remove Annotations from PDF in Python
- Download the updated file
Upload the Document
Firstly, we will upload the PDF file to the cloud using the code sample given below:
As a result, the uploaded file will be available in the files section of the dashboard on the cloud.
Remove Annotations from PDF in Python
Now, we will remove the annotations from the PDF document programmatically by following the steps given below:
- Firstly, create an instance of AnnotateApi.
- Next, create an instance of the FileInfo.
- Then, set the input PDF file path.
- Next, create an instance of the RemoveOptions.
- Then, assign FileInfo to RemoveOptions.
- Next, provide annotation IDs in a comma separated array to remove.
- Then, set the output file path.
- After that, create a request by calling the RemoveAnnotationsRequest method with RemoveOptions object.
- Finally, get results by calling the AnnotateApi.remove_annotations() method with RemoveAnnotationsRequest as argument.
The following code sample shows how to remove annotations from the PDF document using a REST API in Python. We just need to mention annotation IDs to be removed from the document. We can get annotation IDs using the extract() method with ExtractRequest as described here.
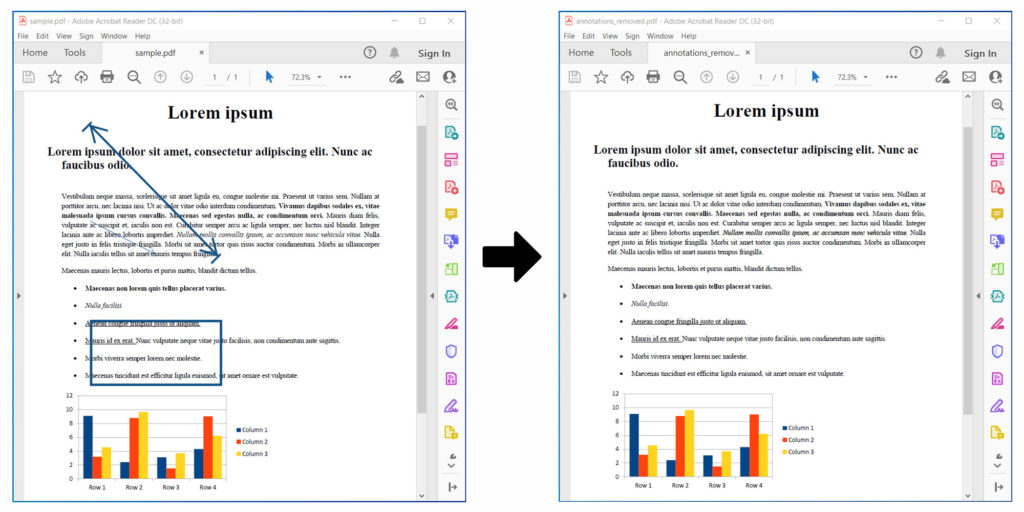
Remove Annotations from PDF in Python.
Download the Output File
The above code sample will save the output file after removing annotations from the PDF file on the cloud. It can be downloaded using the following code sample:
Extract Annotations from PDF Documents in Python
We can extract annotations from the PDF documents programmatically by following the steps given below:
- Firstly, create an instance of AnnotateApi.
- Next, create an instance of the FileInfo.
- Then, set the input file path.
- After that, create a request by calling the ExtractRequest method with the FileInfo object.
- Finally, get results by calling the AnnotateApi.extract() method with ExtractRequest as argument.
The following code sample shows how to extract annotations from the PDF document using a REST API in Python.
The above code sample will return an array of all the annotations in JSON format, as shown below:
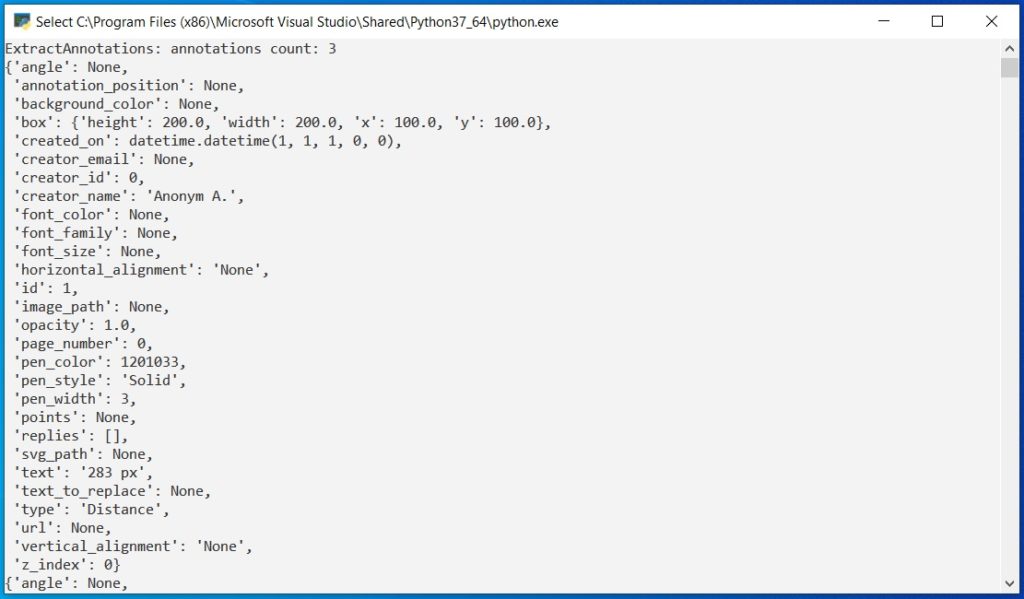
Extract Annotations from PDF Documents in Python.
Try Online
Please try the following free online PDF annotation tool, which is developed using the above API. https://products.groupdocs.app/annotation/pdf
Conclusion
In this article, we have learned how to remove annotations from PDF documents on the cloud. We have also seen how to extract annotations from PDF documents using Python. This article also explained how to programmatically upload a PDF file to the cloud and download the file from the cloud. Besides, you can learn even more about GroupDocs.Annotation Cloud API using the documentation. We also provide an API Reference section that lets you visualize and interact with our APIs directly through the browser. In case of any ambiguity, please feel free to contact us on the forum.