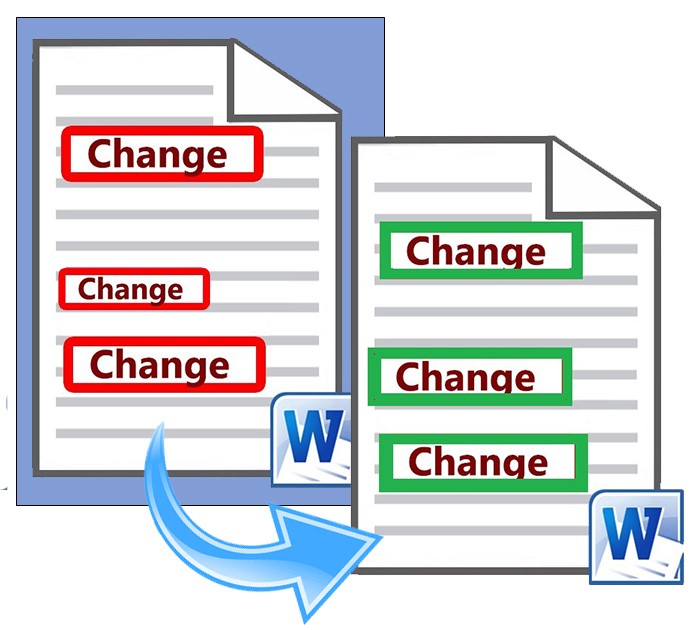
Microsoft Word offers a wonderful feature to track changes and keep revisions for Word documents. As a Python developer, you can accept or reject the tracked changes of Word documents (.docx) programmatically on the cloud. This article will be focusing on how to accept or reject tracked changes of a Word document using a REST API in Python.
The following topics shall be covered in this article:
- Document Comparison REST API and Python SDK
- Accept or Reject Tracked Changes using a REST API in Python
- Accept or Reject All Changes using a REST API in Python
Document Comparison REST API and Python SDK
For working with revisions, I will be using the Python SDK of GroupDocs.Comparison Cloud API. It compares two documents of supported file formats and finds differences between them. As a result, it creates a resultant file containing differences. It also enables you to accept or reject the retrieved changes. You can easily integrate the SDK into your existing Python applications. It empowers you to compare documents, spreadsheets, presentations, Microsoft Visio diagrams, emails, and files of many other formats. It also provides .NET, Java, PHP, Node.js, and Ruby SDKs as its document comparison family members for the Cloud API.
You can install GroupDocs.Comparison Cloud to your Python project using the following command in the console:
pip install groupdocs_comparison_cloud
Please get your Client ID and Client Secret from the dashboard before you start following the steps and available code examples. Once you have your ID and secret, add in the code as demonstrated below:
Accept or Reject Tracked Changes using a REST API in Python
You can accept or reject specific revisions of Word documents by following the simple steps mentioned below:
- Upload the DOCX files to the Cloud
- Accept or Reject Changes using Python
- Download the resultant file
Upload the Document
Firstly, upload the Word document with revisions to the Cloud using the code example given below:
As a result, the uploaded DOCX file will be available in the files section of your dashboard on the cloud.
Accept or Reject Changes using Python
Please follow the steps mentioned below to accept or reject revisions programmatically.
- Create an instance of ReviewApi
- Set the source .docx file
- Define ApplyRevisionsOptions
- Assign source and set the output file
- Create GetRevisionsRequest
- Get revisions by calling the ReviewApi.get_revisions() method
- Set revision action to “Accept” or “Reject” for each revision
- Assign updated revisions to ApplyRevisionsOptions
- Create ApplyRevisionsRequest
- Get results by calling the ReviewApi.apply_revisions() method
The following code example shows how to accept tracked changes using a REST API.
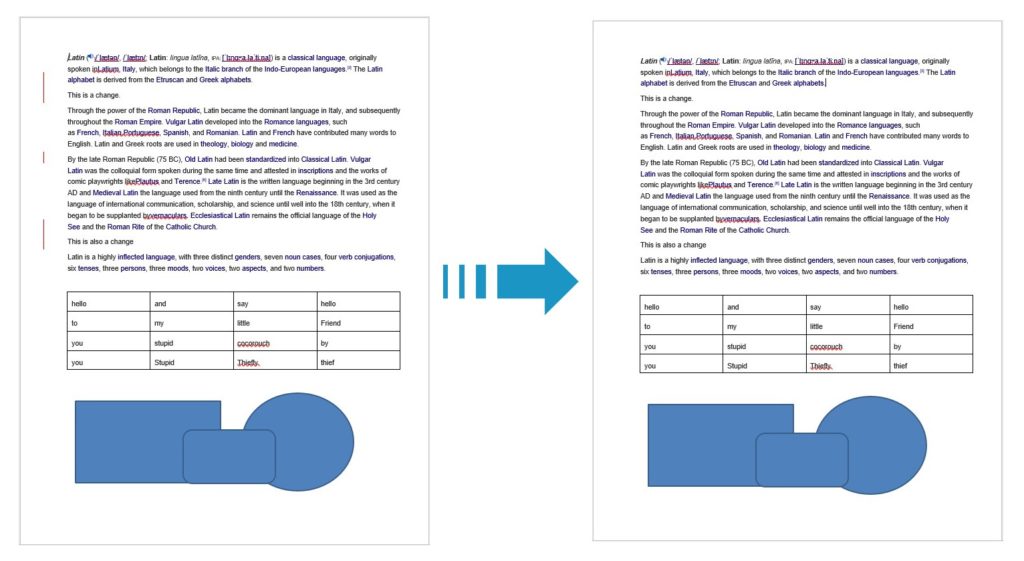
Accept Changes using Python
In case of rejecting any changes, you may use the following code example:
for revision in revisions:
revision.action = "Reject"
Download the Resultant File
As a result, the above code example will save a newly created DOCX file with changes on the cloud. You can download it using the following code sample:
Accept or Reject All the Changes using Python
Please follow the steps mentioned below to accept or reject all the changes at once programmatically.
- Create an instance of ReviewApi
- Set the source .docx file
- Define ApplyRevisionsOptions
- Then assign the source and set the output file
- Set accept_all to “True” for accepting all the changes
- Or set reject_all to “True” for rejecting all the changes
- Then assign updated revisions to ApplyRevisionsOptions
- Create ApplyRevisionsRequest
- Get results by calling the ReviewApi.apply_revisions() method
The following code example shows how to accept all the changes using a REST API. Please follow the steps mentioned earlier to upload and download the file.
You may reject all the revisions by using the following code example:
options.reject_all = True
Try Online
Please try the following free online Word comparison tool, which is developed using the above API. https://products.groupdocs.app/comparison/docx
Conclusion
In this article, you have learned how to accept or reject tracked changes of Microsoft Word documents on the cloud using Python. You also learned how to programmatically upload the DOCX file on the cloud and then download the resulting file from the cloud. You can learn more about GroupDocs.Comparison Cloud API using the documentation. We also provide an API Reference section that lets you visualize and interact with our APIs directly through the browser. In case of any ambiguity, please feel free to contact us on the forum.