As a Python developer, you can compare two or more Word documents (.docx) for similarities and differences programmatically on the cloud. The document comparison helps you track changes in the Word documents. This article will be focusing on how to compare two or more Word documents using a REST API in Python.
The following topics shall be covered in this article:
- Document Comparison REST API and Python SDK
- Compare Word Documents using a REST API
- Compare Multiple Word Documents using Python
Document Comparison REST API and Python SDK
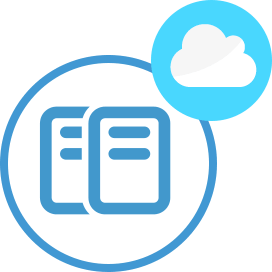
For comparing Microsoft Word documents, I will be using the Python SDK of GroupDocs.Comparison Cloud API. It compares two documents of supported file formats and finds differences between them. As a result, it creates a resultant file containing differences and enables you to accept or reject the retrieved changes. It can easily be integrated into your existing Python applications, to empower your end-users to compare documents, spreadsheets, presentations, Microsoft Visio diagrams, emails, and files of many other formats. It also provides .NET, Java, PHP, and Ruby SDKs as its document comparison family members for the Cloud API.
You can install GroupDocs.Comparison Cloud to your Python project with pip (package installer for python) using the following command in the console:
pip install groupdocs_comparison_cloud
Please get your Client ID and Client Secret from the dashboard before you start following the steps and available code examples. Add your ID and secret in the code as demonstrated below:
Compare Word Documents using a REST API in Python
You can compare two Word documents by following the simple steps mentioned below:
- Upload the DOCX files to the Cloud
- Compare Word Files in Python
- Download the resultant file
Upload the Document
First of all, upload the source and target Word documents to the Cloud using the code example given below:
As a result, the uploaded DOCX files (source.docx, target.docx) will be available in the files section of your dashboard on the cloud.
Compare Word Files in Python
Please follow the steps mentioned below to compare two Word documents programmatically.
- Create an instance of CompareApi
- Set the source .docx file
- Set the target .docx file
- Define ComparisonOptions
- Create ComparisonsRequest
- Get results by calling the CompareApi.comparisons() method
The following code snippet shows how to compare two Word documents using a REST API.
You may provide the password for the password-protected source or target files as shown below:
target.password = "5784"
You may also define various settings to be applied during comparison and assign them to ComparisonOptions as shown below:
Download the Resultant File
The above code sample will save the differences in a newly created DOCX file on the cloud. You can download it using the following code sample:
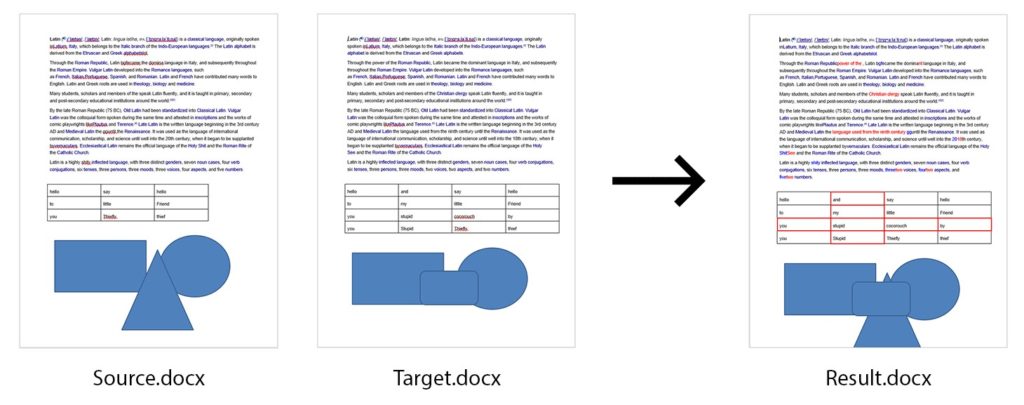
The resultant file also contains a summary page at the end of the document as shown below:
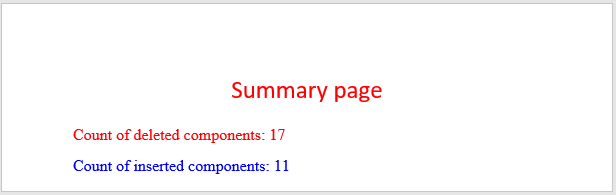
Compare Multiple Word Files using Python
Please follow the steps mentioned below to compare multiple Word documents using Python.
- Create an instance of CompareApi
- Set the source .docx file
- Set multiple target .docx files
- Define _ComparisonOptions _if required
- Create ComparisonsRequest
- Get results by calling the CompareApi.comparisons() method
The following code snippet shows how to compare multiple Word documents using Python. Please follow the steps mentioned earlier to upload multiple DOCX files.
Please try the following free online DOCX comparison tool, which is developed using the above API. https://products.groupdocs.app/comparison/docx
Conclusion
In this article, you have learned how to compare Microsoft Word documents on the cloud with document comparison REST API using Python. You also learned how to programmatically upload two or more files on the cloud and then download the resulting file from the cloud. You can learn more about GroupDocs.Comparison Cloud API using the documentation. We also provide an API Reference section that lets you visualize and interact with our APIs directly through the browser. In case of any ambiguity, please feel free to contact us on the forum.