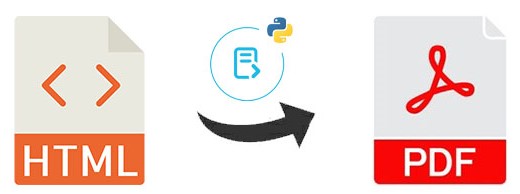
As a Python programmer, you have the capability to programmatically transform your HTML files into PDF documents in a cloud-based environment. This conversion process can prove invaluable for record-keeping purposes and for distributing HTML content in a more portable PDF format. In this guide, we will explore the process of utilizing a REST API in Python to convert HTML into PDF documents.
The following topics shall be covered in this article:
- HTML to PDF Conversion REST API and Python SDK
- Convert HTML to PDF using REST API in Python
- Convert HTML to PDF and Add Watermark
- HTML to PDF Conversion without using Cloud Storage
- Convert HTML to PDF and Download Directly
HTML to PDF Conversion REST API and Python SDK
For converting HTML files to PDF, I will be using the Python SDK of GroupDocs.Conversion Cloud API. It allows you to convert your documents and images of any supported file format to any format you need. You can easily convert between over 50 types of documents and images such as Word, PowerPoint, Excel, PDF, HTML, CAD, raster images, etc.
You can install GroupDocs.Conversion Cloud to your Python project using the following command in the console:
pip install groupdocs_conversion_cloud
Please get your Client ID and Client Secret from the dashboard before you start following the steps and available code examples. Once you have your ID and secret, please add in the code as shown below:
Convert HTML to PDF using REST API in Python
You can convert your HTML file into PDF documents by following the simple steps mentioned below:
- Upload the HTML file to the Cloud
- Convert HTML to PDF in Python
- Download the converted file
Upload the Document
Firstly, upload the HTML file to the cloud using the code example given below:
As a result, the uploaded HTML file will be available in the files section of your dashboard on the cloud.
Convert HTML to PDF in Python
You can easily convert HTML to PDF documents programmatically by following the steps given below:
- Create an instance of ConvertApi
- Create an instance of the ConvertSettings
- Set the HTML file path
- Assign “pdf” to the format
- Provide the output file path
- Define PdfConvertOptions if required
- Optionally set various properties such as dpi, margin_top, margin_left, fit_window, etc.
- Create ConvertDocumentRequest with ConvertSettings
- Get results by calling the convert_document() method with ConvertDocumentRequest
The following code example shows how to convert your HTML file to a PDF document using a REST API.

Convert HTML to PDF using REST API in Python
Download the Converted File
The above code sample will save the converted PDF file on the cloud. You can download it using the following code sample:
Convert HTML to PDF and Add Watermark
You can convert HTML to PDF documents and add watermarks to converted documents programmatically by following the steps given below:
- Create an instance of the ConvertApi
- Create an instance of the ConvertSettings
- Set the HTML file path
- Assign “pdf” to the format
- Provide the output file path
- Create an instance of the WatermarkOptions
- Set Watermark Text, Color, Width, Height, etc.
- Define the PdfConvertOptions and assign WatermarkOptions
- Create ConvertDocumentRequest with ConvertSettings
- Convert by calling the convert_document() method with ConvertDocumentRequest
The following code example shows how to convert an HTML file to a PDF document and add a watermark to the converted PDF document using a REST API in Python. Please follow the steps mentioned earlier to upload and download files.
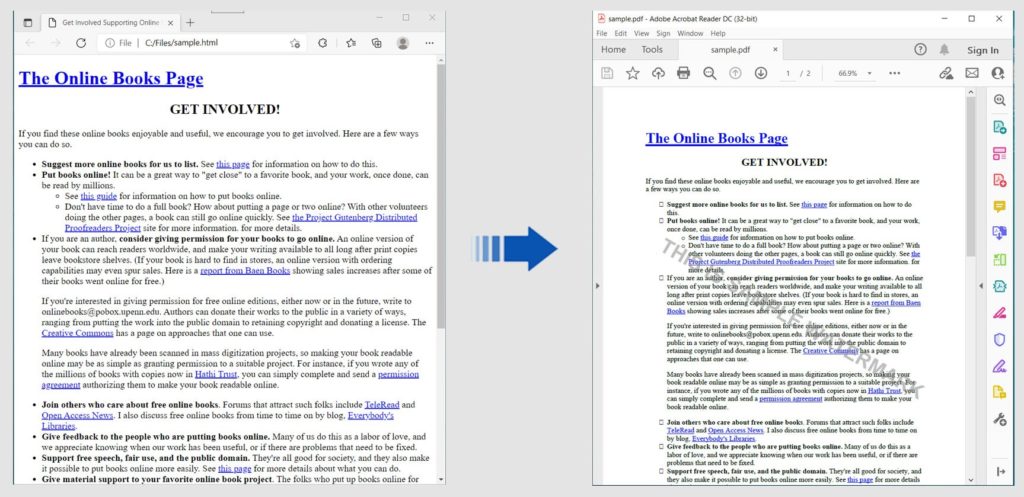
Convert HTML to PDF and Add Watermark
HTML to PDF Conversion without using Cloud Storage
You can convert HTML to PDF documents without using cloud storage by following the steps given below:
- Create an instance of the ConvertApi
- Create ConvertDocumentDirectRequest and pass requested document format and the input file path
- Get results by calling the convert_document_direct_()_ method with ConvertDocumentDirectRequest
- Optionally, save the output file to the local path using shutil.move() method
The following code example shows how to convert an HTML file to a PDF document without using cloud storage. You will pass the input file in the request body and receive the output file in the API response.
Convert HTML to PDF and Download Directly
You can easily convert HTML to PDF documents programmatically by following the steps given below:
- Create an instance of ConvertApi
- Create an instance of the ConvertSettings
- Set the HTML file path
- Assign “pdf” to the format
- Set “None” to the output path
- Create ConvertDocumentRequest with ConvertSettings
- Get results by calling the convert_document_download() method
- Optionally, save the output file to the local path using shutil.move() method
The following code example shows how to convert an HTML file to a PDF document and download it directly using a REST API in Python. The API shall return the converted PDF file in response. Please follow the steps mentioned earlier to upload a file.
Try Online
Please try the following free online HTML to PDF conversion tool, which is developed using the above API. https://products.groupdocs.app/conversion/html-to-pdf
Conclusion
In this article, you’ve gained insights into several valuable aspects. First, you’ve discovered the process of converting HTML files into PDF documents using Python in a cloud-based environment. Additionally, you’ve learned how to perform this conversion without relying on cloud storage, enabling programmatic flexibility.
Furthermore, you’ve acquired knowledge on enhancing PDF documents by adding watermarks using Python. The article has also elucidated the step-by-step procedure for programmatically uploading an HTML file to the cloud and subsequently downloading the converted PDF file.
For a deeper understanding and further exploration, the documentation for GroupDocs.Conversion Cloud API is available. Additionally, we offer an interactive API Reference section, allowing you to visualize and interact with our APIs directly from your browser.
If you encounter any uncertainties or require assistance, please do not hesitate to reach out to our community on the forum. We are here to provide support and clarification.