
You can convert images of popular formats such as JPG, PNG into PDF documents programmatically on the cloud. As a Node.js developer, you can easily convert images into PDF files in your Node.js applications. This article will be focusing on how to convert JPG to PDF using a REST API in Node.js.
The following topics shall be covered in this article:
- Document Conversion REST API and Node.js SDK
- Convert Images to PDF using a REST API in Node.js
- JPG to PDF Conversion with Advanced Options
- Convert JPG to PDF without using Cloud Storage
- Convert JPG to PDF and Add Watermark
Document Conversion REST API and Node.js SDK
I will be using the Node.js SDK of GroupDocs.Conversion Cloud API for converting JPG to PDF. The API allows you to convert your documents to any format you need. It supports the conversion of over 50 types of documents and images such as Word, Excel, PowerPoint, PDF, HTML, JPG, PNG, CAD. It also provides .NET, Java, PHP, Ruby, Android, and Python SDKs as its document conversion family members for the Cloud API.
You can install GroupDocs.Conversion Cloud to your Node.js applications using the following command in the console:
npm install groupdocs-conversion-cloud --save
Please get your Client ID and Client Secret from the dashboard before you start following the steps and available code examples. Once you have your ID and secret, add in the code as shown below:
Convert Images to PDF using a REST API in Node.js
You can convert images to PDF documents by following the simple steps given below:
- Upload the JPG image file to the Cloud
- Convert JPG to PDF using Node.js
- Download the converted PDF file
Upload the Image
Firstly, upload the JPG file to the Cloud using the following code sample:
As a result, the uploaded JPG file will be available in the files section of your dashboard on the cloud.
Convert JPG to PDF using Node.js
Please follow the steps mentioned below to convert JPG to PDF document programmatically:
- Create an instance of ConvertApi
- Create ConvertSettings instance
- Set the JPG file path
- Assign “pdf” to format
- Provide output file path
- Create ConvertDocumentRequest
- Get results by calling the ConvertApi.convertDocument() method
The following code example shows how to convert your JPG image to a PDF document using a REST API in Node.js.

Convert JPG to PDF using Node.js
Download the Converted File
The above code sample will save the converted PDF file on the cloud. You can download it using the following code sample:
JPG to PDF Conversion with Advanced Options
Please follow the steps mentioned below to convert JPG to PDF document with some advanced settings:
- Create an instance of ConvertApi
- Create ConvertSettings instance
- Set the JPG file path
- Assign “pdf” to format
- Provide output file path
- Define PdfConvertOptions
- Set various convert settings such as dpi, imageQuality, height, margins (top, left, right, bottom), etc.
- Create ConvertDocumentRequest
- Get results by calling the ConvertApi.convertDocument() method
The following code example shows how to convert JPG to a PDF document with advanced convert options.
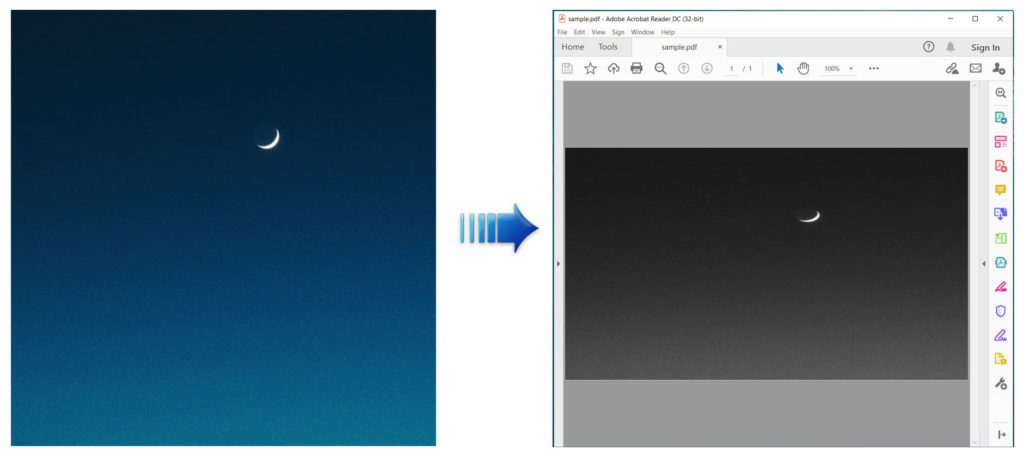
JPG to PDF Conversion with Advanced Options
Convert JPG to PDF without using Cloud Storage
Please follow the steps mentioned below to convert JPG to PDF document without using Cloud storage:
- Create an instance of ConvertApi
- Create ConvertDocumentDirectRequest
- Provide the input file path and target format as input parameters
- Get results by calling the convertDocument_Direct() method
- Save output file to the local path using FileStream.writeFile() method
The following code example shows how to convert JPG to a PDF document without using cloud storage. It means you will pass the input file in the request body and receive the output file in the API response.
Convert JPG to PDF and Add Watermark
Please follow the steps mentioned below to convert JPG to PDF document and then add watermark to the converted PDF:
- Create an instance of ConvertApi
- Create ConvertSettings instance
- Set the JPG file path
- Assign “pdf” to format
- Provide output file path
- Define WatermarkOptions
- Set Watermark Text, Color, Width, Height, etc.
- Define PdfConvertOptions and assign WatermarkOptions
- Create ConvertDocumentRequest
- Get results by calling the ConvertApi.convertDocument() method
The following code example shows how to convert JPG to a PDF document and add a watermark to the converted PDF document using a REST API in Node.js.
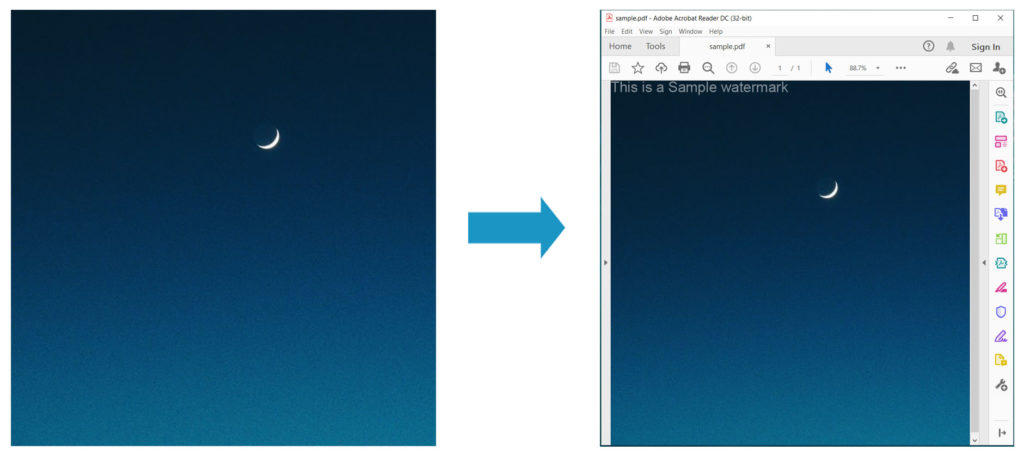
Convert JPG to PDF and Add Watermark
Try Online
Please try the following free online JPG conversion tool, which is developed using the above API. https://products.groupdocs.app/conversion/
Conclusion
In this article, you have learned how to convert JPG to PDF documents on the cloud. You have also learned how to add a watermark to the converted PDF document using Node.js. Furthermore, you have learned how to programmatically upload the JPG file on the cloud and then download the converted file from the cloud. You can learn more about GroupDocs.Conversion Cloud API using the documentation. We also provide an API Reference section that lets you visualize and interact with our APIs directly through the browser. In case of any ambiguity, please feel free to contact us on the forum.