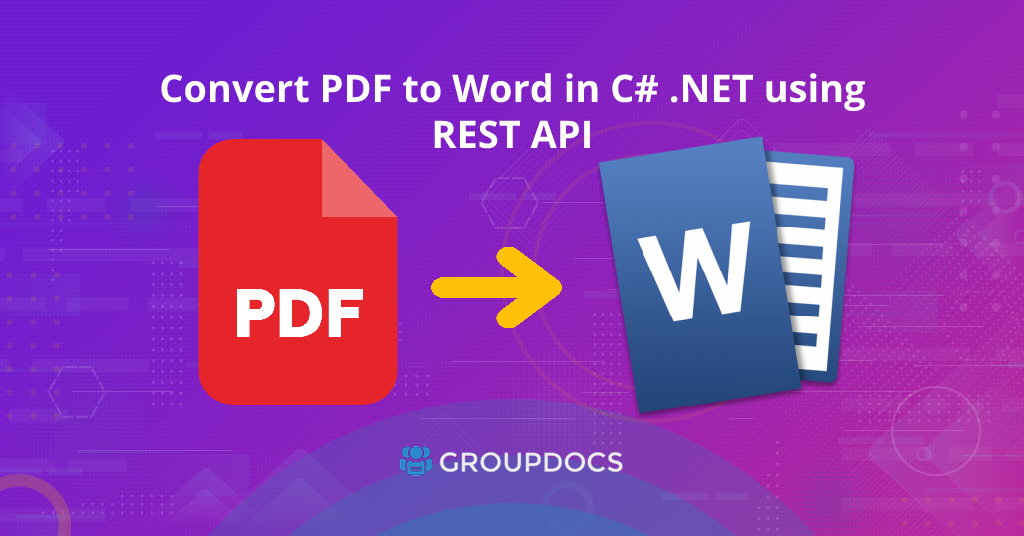
Convert PDF to Word in C# .NET using REST API
PDF (Portable Document Format) is one of the most popular file formats to protect and secure documents online. Word (.doc, .docx) is one of the most commonly used word-processing document formats. It allows you to create, edit, view, and share your documents quickly and easily using the Word processing application. In various cases, you want to convert PDF file to Word file to edit and update documents. So, in this article, I will show you how to convert PDF to Word in C# .NET using REST API.
The following topics shall be covered in this article:
- File and Document Conversion API – .NET SDK Installation
- Convert PDF to Editable Word Document Programmatically in C#
- Convert PDF to Word DOCX in C# using Advanced Options
- How to Convert Range of Pages from PDF to DOCX File in C#
- How to Convert Specific Pages of PDF to Word Document in C#
File and Document Conversion API – .NET SDK Installation
In order to convert PDF to Word Doc, I will be using the .NET SDK of GroupDocs.Conversion Cloud API. It is a fast secure, feature-rich, and reliable file format conversion platform. C# .NET API can convert back and forth between over 50 types of files, including all formats like PDF, HTML, CAD, raster images, and many more. It also allows you to convert and extract format-specific information from a wide list of supported source document formats into any supported document format. Additionally, it provides a flexible set of settings to customize the conversion process. Currently, it supports Java, PHP, Ruby, Python, CSharp,and Node.js SDKs as its document conversion family members
You can download and install it to your VS Code project from the NuGet Package manager or add it using the following command in the Package console:
dotnet add package GroupDocs.Conversion-Cloud --version 22.10.0
Next, get the Client ID and Client Secret from the dashboard before you start following the steps and available code snippets. Add your Client ID and Client Secret in the code as demonstrated below:
Convert PDF to Editable Word Document Programmatically in C#
Converting a PDF to a Word document can be useful when you want to reuse or edit the content of the PDF, or when you want to make it easier to collaborate on the document. You can convert PDF to Word file in CSharp using REST API by following the simple steps mentioned below:
- Upload the PDF document to the Cloud
- Convert PDF file to Word DOCX using REST API
- Download the converted file
Upload the PDF File
Firstly, upload the PDF document to the Cloud using any of the following methods:
- Using the dashboard
- Upload source file using Upload File API from the browser
- Upload programmatically using the code example given below:
As the result, the PDF file will be uploaded to the cloud storage.
Convert PDF to Word File Online
This section demonstrates how to convert PDF files to Word files programmatically in C# using REST API. Follow the steps mentioned below:
- Firstly, create an instance of the ConvertApi
- Secondly, create an instance of the ConvertSettings
- Next, provide the input PDF file path
- Then, assign “docx” to the format
- Create an instance of the PdfLoadOptions
- Provide the input file password
- Now, set the output file path
- Create ConvertDocumentRequest with ConvertSettings
- Finally, convert by calling the convertDocument() method with ConvertDocumentRequest
The following code example shows how to convert PDF to Word DOCX in C# using REST API:
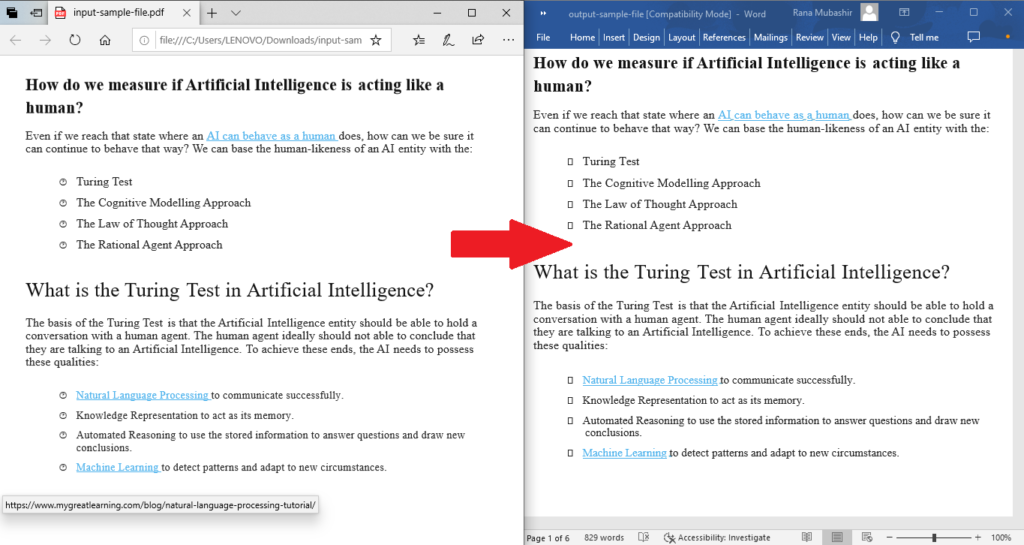
Convert PDF to Word DOCX
Download the Converted File
The above code sample will save the converted Word file on the cloud. You can download it using the following code sample:
Convert PDF to Word DOCX in C# using Advanced Options
Next, convert PDF file to Word document using additional settings by following the steps mentioned below:
- Firstly, create an instance of the ConvertApi
- Secondly, create an instance of the ConvertSettings
- Next, provide the PDF file path as input
- Then, assign “docx” to the format
- Now, create an instance of the PdfLoadOptions
- Provide a password for the input file
- Create an instance of the DocxConvertOptions
- Optionally set various convert parameters like Password, Zoom, Dpi, Width, Height, etc.
- Provide the output file path
- Create ConvertDocumentRequest with ConvertSettings
- Lastly, convert by calling the convertDocument() method with ConvertDocumentRequest
The following code example shows how to convert PDF file to Word document with advanced convert options:
How to Convert Range of Pages from PDF to DOCX File in C#
This section is about how to convert selected range of pages from PDF file to Word. So, you have to provide a range of pages as demonstrated in the code snippet below. Convert a range of pages from a PDF file to Word document programmatically by following the steps given below:
- Create an instance of the ConvertApi
- Next, create an instance of the ConvertSettings
- Provide the PDF file path as input
- Now, assign “docx” to the format
- Create an instance of the PdfLoadOptions
- Provide a password for the input file
- Create an instance of the DocxConvertOptions
- Now, set pages range parameters FromPage and PagesCount with the document password.
- Next, provide the output file path
- Create ConvertDocumentRequest with ConvertSettings
- Finally, convert by calling the convertDocument() method with ConvertDocumentRequest
The following code sample shows how to convert a range of pages from PDF to Word DOCX using REST API in C#:
Please follow the steps mentioned earlier to upload and download a file.
How to Convert Specific Pages of PDF to Word Document in C#
In this section, you can convert specific pages of PDF file to Word format programmatically by following the steps mentioned below:
- Create an instance of the ConvertApi
- Create an instance of the ConvertSettings
- Provide the PDF file path as input
- Now, assign “docx” to the format
- Create an instance of the PdfLoadOptions
- Provide a password for the input file
- Create an instance of the DocxConvertOptions
- Now, set the page collection array with the document password.
- Provide the output file path
- Create ConvertDocumentRequest with ConvertSettings
- Finally, convert by calling the convertDocument() method with ConvertDocumentRequest
The following code example shows how to convert specific pages of PDF file to Word document using REST API in C#:
Please follow the steps mentioned earlier to upload and download a file.
Online PDF to Word Converter Free
How to convert PDF to Word online? Please try the following free online PDF to Word converter without changing the format, which is developed using the above API.
Summing up
In this article, you have learned:
- how to convert PDF to Word document in C# using REST API;
- convert selected pages from PDF file to Word DOC in C# using REST API;
- programmatically convert specific pages of PDF to DOCX format in C#;
- programmatically upload the PDF file and download the converted Word file from the cloud;
Additionally, we advise you to refer to our Getting Started guide. We also provide an API Reference section that lets you visualize and interact with our APIs directly through the browser.
Finally, groupdocs.cloud is writing new blog articles on online file converters between multiple file formats. So, please stay in touch for regular updates.
Ask a question
For any queries/discussions about PDF to Word conversion, feel free to visit our forum.
FAQs
How do I convert PDF to Word DOC programmatically?
Please follow this link to learn the C# code snippet for how to convert PDF file to Word document quickly.
How to install PDF to Word converter API?
Install free download C# library to download, process, and convert PDF to Word DOCX format programmatically.
Can I convert PDF to Word for free?
Yes, you can convert PDF to DOC using an online PDF to Word editable converter for free.
What is the best PDF to DOCX Converter?
PDF to Document converter online is the best free PDF to DOCX converter online.
See Also
We recommend you visit the following articles to learn about:
- Convert Word to Markdown and Markdown to Word in Python
- Convert Markdown to PDF and PDF to Markdown in Python
- How to Convert EXCEL to JSON and JSON to EXCEL in Python
- How to Convert PDF to Editable Word Document using Node.js
- Convert Word Documents to PDF using REST API in Python
- How to Convert PDF to Excel in Python using REST API
- Convert CSV to JSON and JSON to CSV in Python
- Convert PowerPoint PPT/PPTX to JPG/JPEG Images in Python
- Programmatically Convert HTML to PDF using REST API in Python
- Programmatically Convert Excel to CSV using REST API in Python