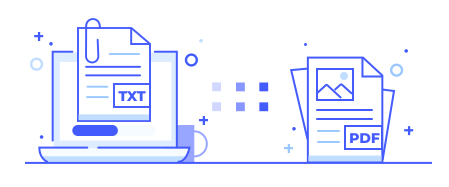
Convert Text Files to PDF using File Conversion API in Python
Notepad is windows text editor and word processing program to create quick notes in a text file while PDFs are one of the most important and widely used digital media. Converting text or txt file to PDF document is one of the basic requirements in real life. Online Text to PDF is used to present and exchange documents reliably, independent of software, or operating system. To convert TXT files to PDF programmatically, this article demonstrates how to convert Text files to PDF using file conversion API in Python.
The following topics shall be covered in this article:
- Text to PDF Conversion REST API and Python SDK
- How to Convert Text to PDF using REST API in Python
- Convert Text to PDF with Advanced Options in Python
- Convert Range of Pages from Text to PDF in Python
- Convert Specific Pages of Text to PDF in Python
Text to PDF Conversion REST API and Python SDK
For converting Text to PDF, we will be using the Python SDK of GroupDocs.Conversion Cloud API. It is a platform independent documents and images conversion solution. It allows you to quickly and reliably convert images and documents of any supported file format to any format you need.
You can install GroupDocs.Conversion Cloud to your Python application using the following command in the console:
pip install groupdocs_conversion_cloud
Please get your Client ID and Secret from the dashboard before following the mentioned steps. Once you have your ID and secret, add in the code as shown below:
How to Convert Text to PDF using REST API in Python
You can convert your text files to PDF programmatically on the cloud by following the simple steps given below:
Upload the TXT File
Firstly, upload the text file to the cloud using the following code sample:
As a result, the uploaded text file will be available in the files section of your dashboard on the cloud.
Convert TXT to PDF using Python
You can easily convert TXT to PDF document programmatically by following the steps mentioned below:
- Firstly, create an instance of the ConvertApi
- Now, create an instance of the ConvertSettings
- Then, provide the input text file path
- Set output file format as the “pdf”
- Next, provide the output file path
- Now, create ConvertDocumentRequest with ConvertSettings
- Finally, convert text file by calling the convert_document() method with ConvertDocumentRequest.
The following code example shows how to convert TEXT to PDF using REST API in Python:
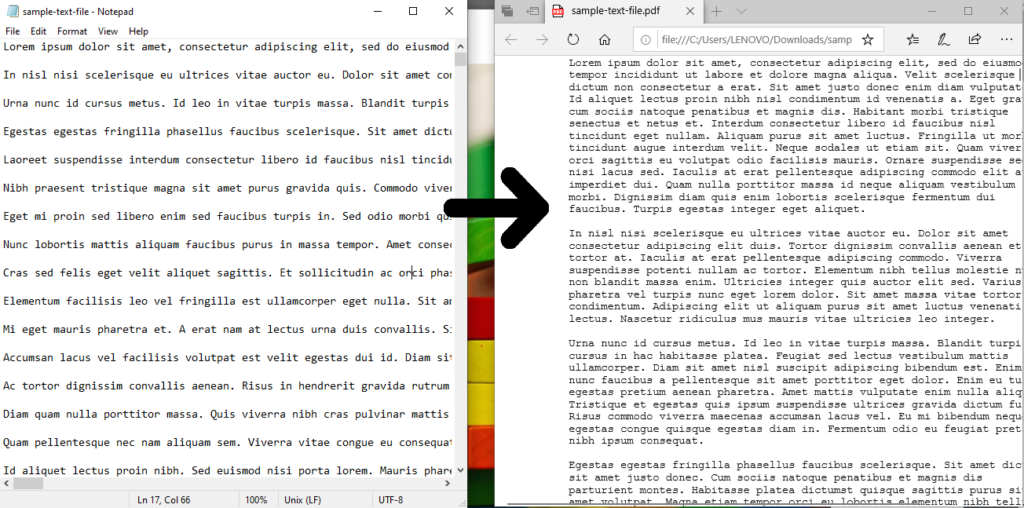
Convert Text to PDF using REST API in Python.
Download the Converted File
The above code sample will save the converted PDF file on the cloud. You can download it using the following code sample:
Convert Text to PDF with Advanced Options in Python
You can convert text documents to PDF files using advanced settings by following the steps given below:
- Firstly, create an instance of the ConvertApi.
- Now, create an instance of the ConvertSettings.
- Then, provide the text file path.
- Next, set the “pdf” as format.
- Now, provide the output file path.
- Now, create an instance of the TextLoadOptions
- Optionally set various load options such as encoding etc.
- Now, create an instance of the PdfConvertOptions
- Then, set various convert options such as center_window, display_doc_title, margins (top, left, right, bottom), etc.
- Next, set convert_options value with pdf convertOptions
- Now, create ConvertDocumentRequest with ConvertSettings
- Finally, convert text by calling the convert_document() method with ConvertDocumentRequest
The following code example shows how to convert text file to PDF document using advanced options. Please follow the steps mentioned earlier to upload and download files from the cloud:
Convert Range of Pages from Text to PDF in Python
You can convert a range of pages from text file to PDF file programmatically by following the steps mentioned below:
- Firstly, create an instance of the ConvertApi
- Now, create an instance of the ConvertSettings
- Then, provide the input text file path
- Assign “pdf” to the format
- Provide the output file path
- Now, create an instance of the PdfConvertOptions
- Then, provide a page range to convert from start page number and total pages to convert
- Now, assign _PdfConvertOptions_ to ConvertSettings
- Then, create ConvertDocumentRequest with ConvertSettings
- Finally, convert by calling the convert_document() method with ConvertDocumentRequest
The following code sample shows how to convert a range of pages from TXT to PDF document using REST API in Python. Please follow the steps mentioned earlier to upload and download resultant pdf file:
Convert Specific Pages of Text to PDF in Python
You can convert specific pages of a text document to a PDF file programmatically by following the steps mentioned below:
- Firstly, create an instance of the ConvertApi
- Now, create an instance of the ConvertSettings
- Then, provide the input text file path
- Assign “pdf” to the format
- Provide the output file path
- Now, create an instance of the PdfConvertOptions
- Then, provide specific page numbers in a comma-separated array to convert
- Now, assign _PdfConvertOptions_ to ConvertSettings
- Then, create ConvertDocumentRequest with ConvertSettings
- Finally, convert by calling the convert_document() method with ConvertDocumentRequest
The following code example shows how to convert specific pages of text file to PDF using REST API in Python. Please follow the steps mentioned earlier to upload and download output pdf file:
Try Online
Do you want to convert text to pdf online? Please try the following free text to pdf converter online, which is developed using the above API. You can easily convert text to pdf online free using this text to pdf maker online.
Summing up
In this article, you have learned:
- how to convert plain text to PDF documents on the cloud;
- how to programmatically upload the text file using python;
- how to download the converted PDF file from the cloud in python;
- how to convert specific pages or a range of pages from text notepad to PDF in Python;
Besides, you can learn more about GroupDocs.Conversion Cloud API using the documentation. We also provide an API Reference section that lets you visualize and interact with our APIs directly through the browser.
Ask a question
If you have any questions about how to change text file to pdf, please feel free to ask in Free Support Forum and it will be answered within a few hours.
See Also
- Convert HTML to PDF using REST API in Python
- How to Convert PDF to JPG, PNG, BMP, TIFF Image Formats
- How to Convert PDF to HTML using REST API in Ruby
- Converting Word to Image Formats using REST API in Ruby
- Convert PowerPoint to PDF using File Conversion API
- Convert PDF to Editable Word Document using Ruby