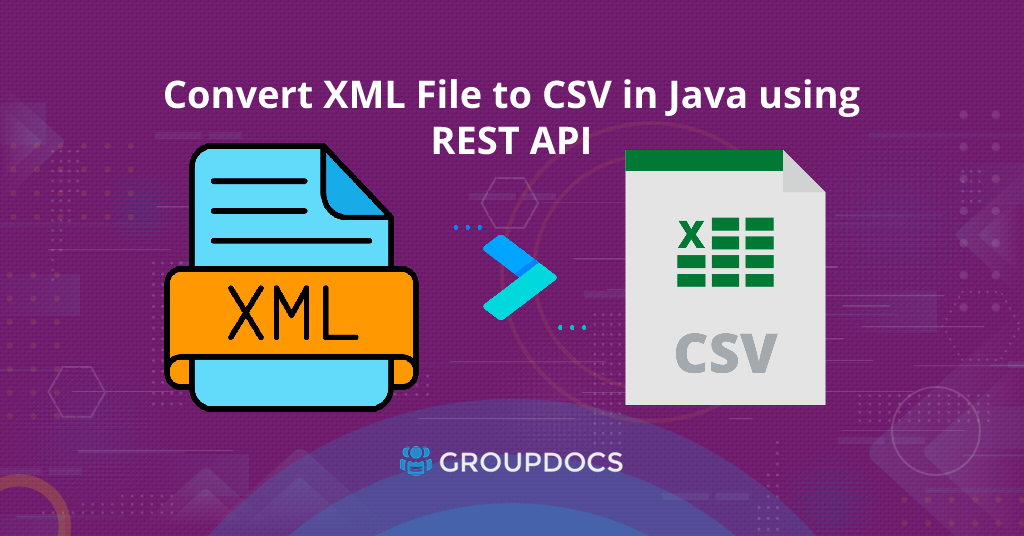
Convert XML file to CSV in Java using REST API.
XML (eXtensible Markup Language) is a markup language for storing, transmitting, and reconstructing data between different applications. CSV (Comma Separated Values), on the other hand, is a simple file format designed to store tabular data. Sometimes, it can be difficult to manage when dealing with large amounts of data. Because of that many developers prefer to convert XML files to CSV format. XML to CSV conversion simplifies the data into a tabular format that is easy to manage and read. So, this article will demonstrate how to convert XML files to CSV in Java using REST API.
We will cover the following topics in this article:
- Java XML to CSV Conversion REST API and SDK Installation
- How to Convert XML File to CSV in Java using REST API
Java XML to CSV Conversion REST API and SDK Installation
GroupDocs.Conversion Cloud SDK for Java is a cloud-based document conversion solution that enables developers to convert various document formats to other formats programmatically in Java. It allows you to convert your documents, images, and email messages of any supported file format to any format with ease. This RESTful API can be integrated into Java applications to provide fast and reliable conversion capabilities.
You can either download the API JAR file or install API using Maven configurations. Add repository and dependency into your project’s pom.xml file.
Maven Repository:
<repository>
<id>groupdocs-artifact-repository</id>
<name>GroupDocs Artifact Repository</name>
<url>https://repository.groupdocs.cloud/repo</url>
</repository>
Maven Dependency:
<dependency>
<groupId>com.groupdocs</groupId
<artifactId>groupdocs-conversion-cloud</artifactId>
<version>23.4</version>
<scope>compile</scope>
</dependency>
Now, sign up for a GroupDocs account and get your API key. Once you have the Client Id and Client Secret, add below code snippet in a Java-based application:
How to Convert XML File to CSV in Java using REST API
Once the installation process is completed, you can jump to the code snippet that converts XML files to CSV format programmatically. Here’s how you can convert XML files to CSV in Java using GroupDocs.Conversion Cloud REST API:
Upload the File
Firstly, upload the XML file to the cloud using the code snippet given below:
As a result, the uploaded XML file will be available in the files section of your dashboard on the cloud.
Convert XML to CSV File in Java
In this section, we will see how to convert an XML file to CSV format programmatically in a Java application. You may follow the steps and the code snippet mentioned below:
- Firstly, import the required classes into your Java file.
- Secondly, create an instance of the ConvertApi class.
- Thirdly, create an instance of ConvertSettings class.
- Next, provide your cloud storage name.
- Now, set the source file path and the target format to “csv”.
- After that, set the output file path.
- Then, create the ConvertDocumentRequest class instance and pass the settings parameter.
- Finally, convert XML to CSV by calling the convert_document() method and passing the ConvertDocumentRequest parameter.
The following code snippet demonstrates how to convert XML to CSV file in Java using REST API:
You can see the output in the image below:

Convert XML to CSV file with Java using REST API
Download the Converted File
The above code sample will save the converted CSV file in the cloud. You can download it using the following code sample:
Free Online XML to CSV Converter
How to convert XML to CSV online for free? Please try the following free online XML to CSV converter. This converter is developed using the above-mentioned GroupDocs.Conversion Cloud REST API.
Conclusion
We can end this blog post here. Hopefully, you have enjoyed the article and learned:
- how to change XML to CSV programmatically in Java;
- programmatically upload XML files and then download the converted CSV file from the cloud;
- and convert any XML file to CSV for free using a free online XML to CSV converter.
In addition, you can learn more about GroupDocs file format conversion API using the documentation, or by examples available on GitHub. We also provide an API Reference section that lets you visualize and interact with our APIs directly through the browser.
Finally, we keep writing new blog articles on different file formats and conversions using REST API. So, please get in touch for the latest updates.
Ask a question
You can ask your queries about how to convert an XML document to a CSV file, via our forum.
FAQs
How do I get started with GroupDocs.Conversion for Java SDK REST API?
You need to sign up for a GroupDocs account and download the GroupDocs.Conversion for Java SDK library, and add it to your Java project.
How can I convert an XML file to a CSV file in Java using REST API?
You need to upload the XML file to the cloud, then convert it to CSV format using the Java code provided. You will also need to download the converted file. The code snippet provided demonstrates the steps to convert an XML file to a CSV file using REST API.
How to convert XML to CSV on Windows?
Please visit the download link to download the XML to CSV offline converter for Windows. This free XML to CSV converter can be used to convert XML documents to CSV files on Windows quickly, with a single click.
How do I convert an XML to a CSV file online for free?
Please follow the step-by-step instructions to convert an XML file to CSV online for free:
- Open online XML to CSV converter.
- Now, click inside the file drop area to upload an XML file or drag & drop an XML file.
- Next, click on the Convert Now button. Online XML to CSV converter will transform XML into a CSV file.
- The download link of the output file will be available instantly after conversion.
See Also
If you want to learn more about related topics, we recommend reading the articles listed below:
- Convert Word to Markdown and Markdown to Word in Python
- Convert PDF to Excel in Python using REST API
- How to Extract Pages From Word Documents in Python
- Convert Word Documents to PDF using REST API in Python
- Convert PDF to Editable Word Document with Python SDK
- How to Convert EXCEL to JSON and JSON to EXCEL in Python
- Convert MSG and EML files to PDF in Python
- Convert XML to CSV and CSV to XML in Python
- How to Convert CSV to JSON and JSON to CSV in Python
- Convert Word to PDF Programmatically in C#
- Convert HTML to PDF in Java using REST API