
In certain cases, we may need to edit Word documents programmatically. We can easily add, edit or delete the content of DOC or DOCX files or apply text formatting using Python. In this article, we will learn how to edit Word documents using a REST API in Python.
The following topics shall be covered in this article:
- Word Document Editor REST API and Python SDK
- Edit Word Document using REST API in Python
- Add Table in Word Documents using Python
- Insert Image in Word Documents using Python
Word Document Editor REST API and Python SDK
For editing the DOCX files, we will be using the Python SDK of GroupDocs.Editor Cloud API. Please install it using the following command in the console:
pip install groupdocs_editor_cloud
Please get your Client ID and Secret from the dashboard before following the mentioned steps. Once you have your ID and secret, add in the code as shown below:
Edit Word Documents using REST API in Python
We can edit Word documents by following the simple steps mentioned below:
Upload the Document
Firstly, we will upload the DOCX file to the Cloud using the code example given below:
As a result, the uploaded DOCX file will be available in the files section of the dashboard on the cloud.
Edit Word Document using Python
We can edit the Word document programmatically by following the steps given below:
- Firstly, create instances of the FileApi and the EditApi.
- Next, create an instance of the FileInfo and provide the input DOCX file path.
- Then, initialize an instance of the WordProcessingLoadOptions and assign FileInfo.
- Next, create the LoadRequest with WordProcessingLoadOptions object as argument.
- Then, call the EditApi.load() method with the LoadRequest object to load the input DOCX file.
- After that, create the DownloadFileRequest with the loaded file.
- Then, call the FileApi.download_file() method to download file as an HTML document.
- Next, read the downloaded HTML file as a string.
- Then, edit the HTML and save the updated HTML document.
- Next, create UploadFileRequest and pass HTML path and file as parameters.
- Then, call the FileApi.upload_file() method with UploadFileRequest to upload the updated HTML file.
- Next, create an instance of the WordProcessingSaveOptions to save in the DOCX.
- After that, create SaveRequest with WordProcessingSaveOptions object.
- Finally, save HTML back to DOCX using the EditApi.save() method with SaveRequest object.
The following code sample shows how to edit a Word document using a REST API in Python.
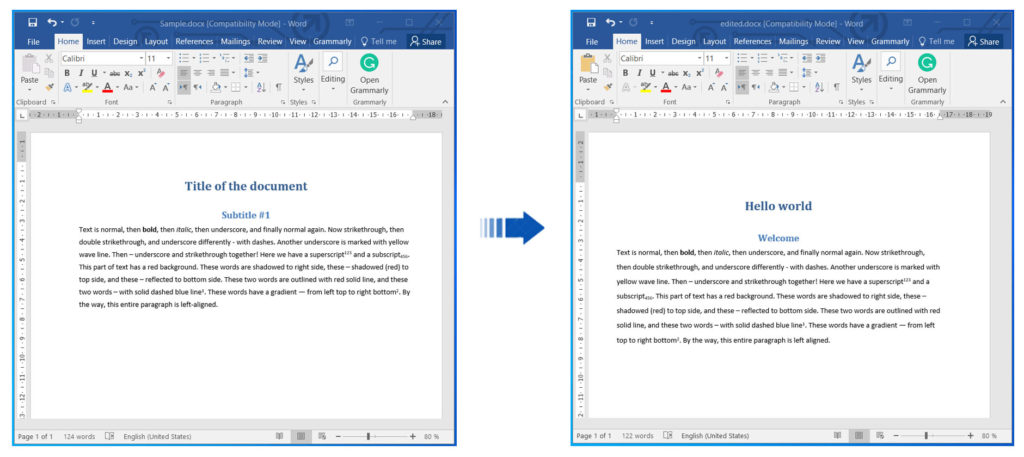
Edit Word Documents using REST API in Python.
Download the Updated File
The above code sample will save the edited Word document (DOCX) on the cloud. It can be downloaded using the following code sample:
Add Table in Word Documents using Python
We can add a table in Word documents programmatically by following the steps mentioned earlier. However, we need to update the HTML to add a table in the document as shown below:
html = html.replace("left-aligned.", """left-aligned. <br/><table style="width: 100%;background-color: #dddddd;">
<caption style=\"font-weight:bold;\"> Persons List</caption>
<tr><th>First Name</th><th>Last Name</th><th>Age</th></tr>
<tr><td>Jill</td><td>Smith</td><td>50</td></tr>
<tr><td>Eve</td><td>Jackson</td><td>94</td></tr>
</table>""")
The following code sample shows how to add a table in a Word document using a REST API in Python. Please follow the steps mentioned earlier to upload and download a file.

Add Table in Word Documents using Python.
Insert Image in Word Documents using Python
We can insert an image in Word documents programmatically by following the steps mentioned earlier. However, we need to update the HTML to insert an image in the document as shown below:
html = html.replace("left-aligned.", """left-aligned. <br/> <img src=\"groupdocs.png\" alt=\"signatures\" style=\"width: 128px; height: 128px;\">""");
The following code sample shows how to insert an image in a Word document using a REST API in Python. Please follow the steps mentioned earlier to upload and download a file.
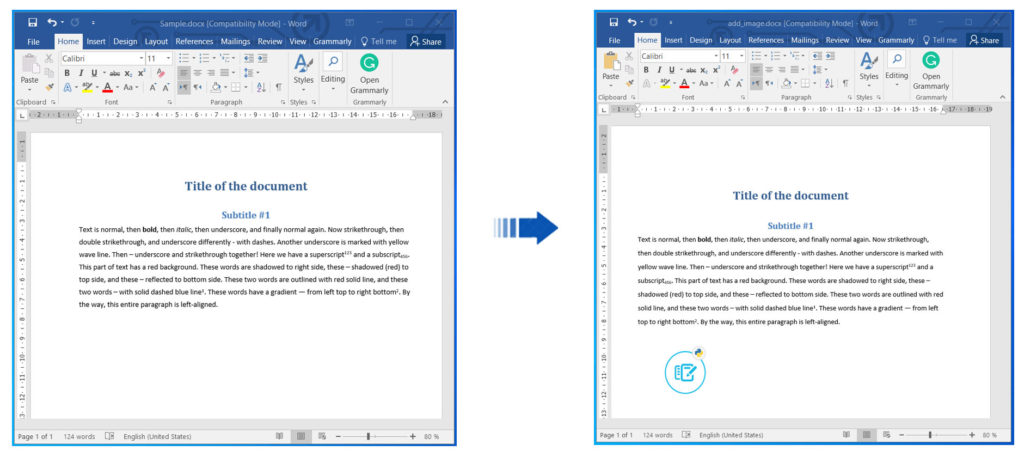
Insert Image in Word Documents using Python.
Try Online
Please try the following free online DOCX editing tool, which is developed using the above API. https://products.groupdocs.app/editor/docx
Conclusion
In this article, we have learned how to edit Word documents on the cloud. We have also seen how to add a table or insert an image in the DOCX file using a REST API in Python. This article also explained how to programmatically upload a DOCX file to the cloud and then download the edited file from the Cloud. Besides, you can learn more about GroupDocs.Editor Cloud API using the documentation. We also provide an API Reference section that lets you visualize and interact with our APIs directly through the browser. In case of any ambiguity, please feel free to contact us on the forum.