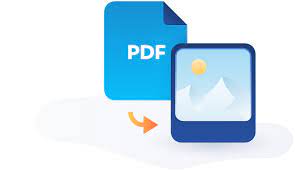
REST API를 사용하여 Node.js에서 PDF를 JPG 이미지로 변환
이미지는 주요 데이터 구성 요소 중 하나이기 때문에 사용자는 PDF를 이미지로 변환해야 하는 경우가 많습니다. 형식의 복잡성으로 인해 PDF 문서의 콘텐츠를 구문 분석하고 표시하는 것은 어렵습니다. 클라우드에서 프로그래밍 방식으로 PDF를 JPG/JPEG와 같은 널리 사용되는 형식의 이미지로 변환할 수 있습니다. Node.js 개발자는 Node.js 애플리케이션에서 온라인으로 PDF를 JPG 고해상도로 쉽게 변환할 수 있습니다. 이 기사는 REST API를 사용하여 node.js에서 PDF를 JPG 이미지로 변환하는 방법에 중점을 둘 것입니다.
이 문서에서는 다음 항목을 다룹니다.
- PDF-이미지 변환기 REST API 및 Node.js SDK
- Node.js에서 REST API를 사용하여 PDF를 JPG 형식으로 변환하는 방법
- 고급 옵션을 사용하여 PDF를 JPG로 변환
- Cloud Storage를 사용하지 않고 PDF를 JPG로 변환
- PDF를 JPG로 변환하고 워터마크 추가
PDF-이미지 변환기 REST API 및 Node.js SDK
PDF를 JPG로 변환하기 위해 GroupDocs.Conversion Cloud의 Node.js SDK API를 사용하려고 합니다. API를 사용하면 문서와 이미지를 필요한 형식으로 변환할 수 있습니다. Word, Excel, PowerPoint, PDF, HTML, JPG, PNG, CAD 등 50가지 이상의 문서 및 이미지 변환을 지원합니다. 또한 .NET, Java, PHP, Ruby, Android 및 Python SDK를 다음과 같이 제공합니다. Cloud API에 대한 문서 변환 제품군.
GroupDocs.Conversion Cloud는 콘솔에서 다음 명령을 실행하여 Node.js 애플리케이션에 설치할 수 있습니다.
npm install groupdocs-conversion-cloud
단계 및 사용 가능한 코드 예제를 시작하기 전에 대시보드에서 클라이언트 ID와 클라이언트 암호를 수집하십시오. ID와 시크릿을 확인한 후 아래와 같이 코드를 추가합니다.
# http://api.groupdocs.cloud에서 노드 애플리케이션의 Node.js SDK 가져오기
global.groupdocs_conversion_cloud = require("groupdocs-conversion-cloud");
global.fs = require("fs");
// https://dashboard.groupdocs.cloud에서 clientId 및 clientSecret을 가져옵니다(무료 등록 필요).
global.clientId = "xxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx";
global.clientSecret = "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx";
global.myStorage = "test-internal-storage";
const config = new groupdocs_conversion_cloud.Configuration(clientId, clientSecret);
config.apiBaseUrl = "https://api.groupdocs.cloud";
Node.js에서 REST API를 사용하여 PDF를 JPG 형식으로 변환하는 방법
이 섹션에서는 PDF 파일을 JPG 이미지로 가져오는 방법에 대한 단계별 가이드를 다룹니다. PDF 파일을 JPG 이미지 파일로 변환하려면 아래의 간단한 단계를 따르십시오.
파일 업로드
먼저 다음 코드 샘플을 사용하여 PDF 파일을 클라우드에 업로드합니다.
// 시스템 드라이브에서 IOStream의 파일을 엽니다.
var resourcesFolder = 'H:\\groupdocs-cloud-data\\sample-file.pdf';
// 파일 읽기
fs.readFile(resourcesFolder, (err, fileStream) => {
// FileApi 구성
var fileApi = groupdocs_conversion_cloud.FileApi.fromConfig(config);
// 업로드 파일 요청 생성
var request = new groupdocs_conversion_cloud.UploadFileRequest("sample-file.pdf", fileStream, myStorage);
// 파일 업로드
fileApi.uploadFile(request)
.then(function (response) {
console.log("Expected response type is FilesUploadResult: " + response.uploaded.length);
})
.catch(function (error) {
console.log("Error: " + error.message);
});
});
결과적으로 업로드된 PDF 파일은 클라우드 대시보드의 파일 섹션에서 액세스할 수 있습니다.
Node.js를 사용하여 PDF를 JPG로 변환
이제 프로그래밍 방식으로 PDF를 JPG 파일로 변환하려면 아래 단계와 코드 샘플을 따르십시오.
- ConvertApi의 인스턴스 생성
- ConvertSettings 클래스의 인스턴스 만들기
- 이제 클라우드 스토리지 이름을 설정하십시오.
- 다음으로 입력 PDF 파일 경로를 설정하십시오.
- 출력 파일 형식을 “jpg"로 설정
- 다음으로 출력 파일 경로를 제공하십시오.
- 이제 ConvertDocumentRequest 클래스의 개체를 만듭니다.
- 마지막으로 ConvertApi.convertDocument() 메서드를 호출하여 출력 파일을 가져옵니다.
다음 코드 예제는 Node.js에서 REST API를 사용하여 품질 손실 없이 PDF를 JPG로 변환하는 방법을 보여줍니다.
// Node.js를 사용하여 PDF 파일을 JPG 이미지 형식으로 변환하는 방법
const convert = async () => {
const convertApi = groupdocs_conversion_cloud.ConvertApi.fromKeys(clientId, clientSecret);
const settings = new groupdocs_conversion_cloud.ConvertSettings();
settings.storageName = myStorage;
settings.filePath = "nodejs-testing/sample-file.pdf";
settings.format = "jpg";
settings.outputPath = "nodejs-testing/sample-file.jpg";
try {
// 변환 문서 요청 생성
const request = new groupdocs_conversion_cloud.ConvertDocumentRequest(settings);
await convertApi.convertDocument(request);
}
catch (err) {
throw err;
}
}
convert()
.then(() => {
console.log("Successfully converted PDF to JPG file format.");
})
.catch((err) => {
console.log("Error occurred while converting the PDF document:", err);
})
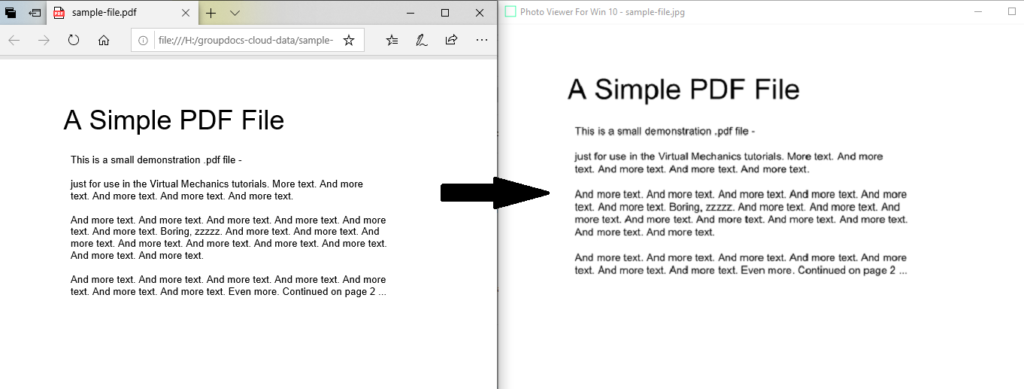
Node.js를 사용하여 PDF를 JPG로 변환
변환된 파일 다운로드
위의 코드 샘플은 변환된 JPG 파일을 클라우드에 저장합니다. 다음 코드 샘플을 사용하여 다운로드할 수 있습니다.
// FileApi를 구성하여 변환된 파일 다운로드
var fileApi = groupdocs_conversion_cloud.FileApi.fromConfig(config);
// 다운로드 파일 요청 생성
let request = new groupdocs_conversion_cloud.DownloadFileRequest("nodejs-testing/sample-file.jpg", myStorage);
// 다운로드 파일 및 응답 유형 스트림
fileApi.downloadFile(request)
.then(function (response) {
// 시스템 디렉토리에 파일 저장
fs.writeFile("H:\\groupdocs-cloud-data\\sample-file.jpg", response, "binary", function (err) { });
console.log("Expected response type is Stream: " + response.length);
})
.catch(function (error) {
console.log("Error: " + error.message);
});
고급 옵션을 사용하여 PDF를 JPG로 변환
다음 단계와 코드 스니펫은 PDF에서 JPG로의 고품질 온라인 변환기 API 및 일부 고급 설정을 사용하여 PDF를 이미지로 변환하는 방법을 보여줍니다.
- ConvertApi 인스턴스 생성
- ConvertSettings 인스턴스 만들기
- 이제 클라우드 스토리지 이름을 설정하십시오.
- 다음으로 PDF 파일 경로를 설정하십시오.
- 그런 다음 “jpg” 값을 형식으로 설정합니다.
- JpgConvertOptions 정의
- grayscale, fromPage, pagesCount, quality, rotateAngle, usePdf 등과 같은 다양한 변환 설정을 지정합니다.
- 이제 대화와 출력 파일 경로를 설정하십시오.
- ConvertDocumentRequest 객체 생성
- 마지막으로 ConvertApi.convertDocument() 클래스를 호출하여 PDF를 JPG로 변환합니다.
다음 코드 예제는 고급 변환 옵션을 사용하여 온라인에서 pdf를 jpg 형식으로 변환하는 방법을 보여줍니다.
// 고급 옵션을 사용하여 PDF를 JPG로 변환하는 방법
const convert_options = async () => {
const convertApi = groupdocs_conversion_cloud.ConvertApi.fromKeys(clientId, clientSecret);
const settings = new groupdocs_conversion_cloud.ConvertSettings();
settings.storageName = myStorage;
settings.filePath = "nodejs-testing/sample-file.pdf";
settings.format = "jpg";
convertOptions = new groupdocs_conversion_cloud.JpgConvertOptions()
convertOptions.grayscale = true;
convertOptions.fromPage = 1;
convertOptions.pagesCount = 1;
convertOptions.quality = 100;
convertOptions.rotateAngle = 90;
convertOptions.usePdf = false;
settings.convertOptions = convertOptions;
settings.outputPath = "nodejs-testing/sample-file.jpg";
try {
// 변환 문서 요청 생성
const request = new groupdocs_conversion_cloud.ConvertDocumentRequest(settings);
await convertApi.convertDocument(request);
}
catch (err) {
throw err;
}
}
convert_options()
.then(() => {
console.log("Converted PDF to JPG image with advanced options.");
})
.catch((err) => {
console.log("Error occurred while converting the PDF file:", err);
})
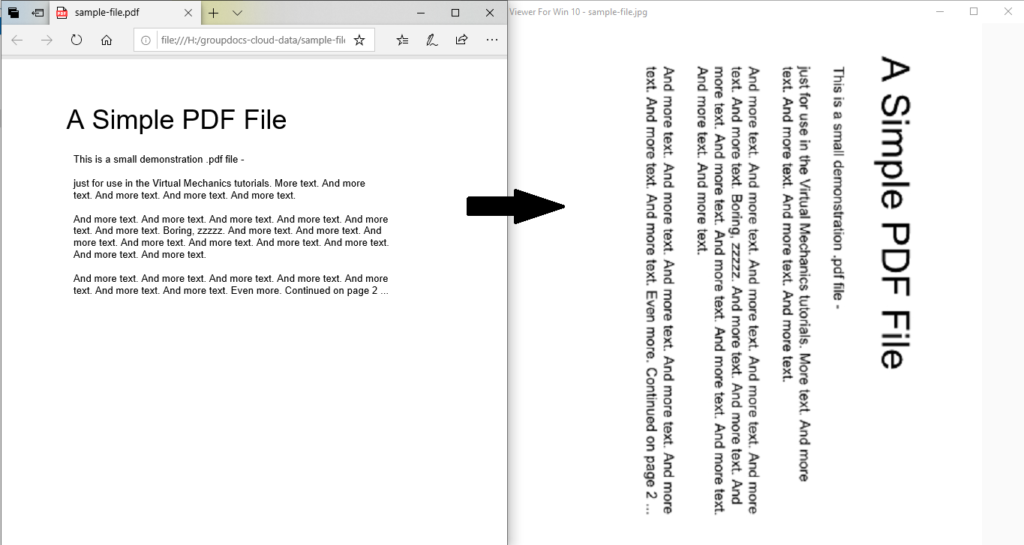
고급 옵션을 사용하여 PDF에서 JPG 단일 이미지로 변환
Cloud Storage를 사용하지 않고 PDF를 JPG로 변환
클라우드 스토리지를 사용하지 않고 PDF를 JPG로 변환하려면 다음 단계를 따르십시오.
- ConvertApi 인스턴스 생성
- ConvertDocumentDirectRequest 만들기
- 입력 파일 경로 및 대상 형식을 입력 매개변수로 제공
- convertDocumentDirect() 메서드를 호출하여 결과 얻기
- FileStream.writeFile() 메서드를 사용하여 출력 파일을 로컬 경로에 저장합니다.
다음 코드 스니펫은 클라우드 스토리지를 사용하지 않고 PDF 문서를 JPG로 변환하는 방법을 보여줍니다. 이는 요청 본문에 입력 파일을 전달하고 API 응답에서 출력 파일을 수신함을 의미합니다.
// Cloud Storage를 사용하지 않고 PDF를 JPG로 변환하는 방법
const convertdirect = async () => {
// API 초기화
const convertApi = groupdocs_conversion_cloud.ConvertApi.fromKeys(clientId, clientSecret);
// 입력 파일
let file = fs.readFileSync('H:\\groupdocs-cloud-data\\sample-file.pdf');
try {
// 변환 문서 요청 생성
const request = new groupdocs_conversion_cloud.ConvertDocumentDirectRequest("jpg", file);
// 문서 변환
let result = await convertApi.convertDocumentDirect(request);
// 로컬 경로에 저장
fs.writeFile("H:\\groupdocs-cloud-data\\sample-file.jpg", result, "binary", function (err) { });
}
catch (err) {
throw err;
}
}
convertdirect()
.then(() => {
console.log("Directly converted PDF to JPG file format.");
})
.catch((err) => {
console.log("Error occurred while directly converting the PDF file:", err);
})
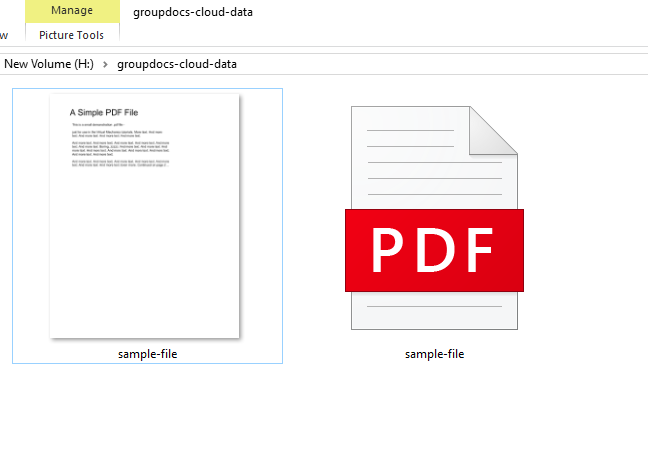
Cloud Storage를 사용하지 않고 여러 pdf를 jpg로 변환
PDF를 JPG로 변환하고 워터마크 추가
다음 단계를 사용하여 PDF를 JPG로 변환한 다음 변환된 PDF에 워터마크를 추가하십시오.
- ConvertApi 인스턴스 생성
- ConvertSettings 개체 만들기
- 이제 클라우드 스토리지 이름을 설정하십시오.
- 다음으로 PDF 파일 경로를 설정하십시오.
- 이제 형식에 “jpg"를 할당합니다.
- 출력 파일 경로 제공
- WatermarkOptions 클래스 정의
- 텍스트, 색상, 너비, 높이, 배경 등과 같은 워터마크 옵션을 설정합니다.
- PdfConvertOptions 정의 및 WatermarkOptions 지정
- 이제 변환 옵션 값을 설정하십시오.
- 그런 다음 ConvertDocumentRequest 인스턴스를 생성합니다.
- 마지막으로 ConvertApi.convertDocument() 클래스를 사용하여 변환하고 호출합니다.
다음 코드 예제는 PDF 문서를 JPG로 변환하고 Node.js에서 REST API를 사용하여 변환된 PDF 문서에 워터마크를 추가하는 방법을 보여줍니다.
// 워터마크가 있는 PDF를 JPG 파일 이미지로 변환하는 방법.
const convertwatermark = async () => {
// API 초기화
const convertApi = groupdocs_conversion_cloud.ConvertApi.fromKeys(clientId, clientSecret);
// 변환 설정 정의
const settings = new groupdocs_conversion_cloud.ConvertSettings();
settings.storageName = myStorage;
settings.filePath = "nodejs-testing/sample-file.pdf";
settings.format = "jpg";
settings.outputPath = "nodejs-testing/sample-file.jpg";
// 워터마크 옵션 정의
let watermark = new groupdocs_conversion_cloud.WatermarkOptions();
watermark.text = "This is a Sample watermark";
watermark.color = "Red";
watermark.width = 250;
watermark.height = 300;
watermark.top = 150;
watermark.left = 300;
watermark.rotationAngle = 45;
watermark.bold = true;
watermark.background = false;
// PDF 변환 옵션 정의
let convertOptions = new groupdocs_conversion_cloud.JpgConvertOptions()
convertOptions.watermarkOptions = watermark;
settings.convertOptions = convertOptions;
try {
// 변환 문서 요청 생성
const request = new groupdocs_conversion_cloud.ConvertDocumentRequest(settings);
await convertApi.convertDocument(request);
}
catch (err) {
throw err;
}
}
convertwatermark()
.then(() => {
console.log("watermark added to converted PDF to JPG image.");
})
.catch((err) => {
console.log("Error occurred while converting the PDF document:", err);
})
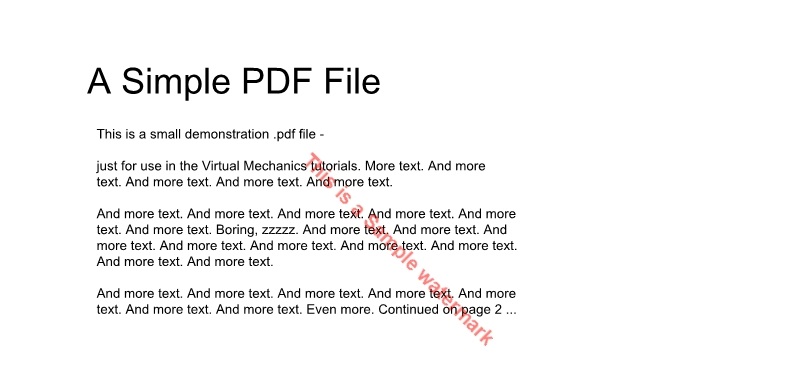
PDF를 JPG로 변환하고 워터마크 추가
온라인 PDF to JPG 변환기 무료
PDF에서 JPG 고품질 변환기를 온라인에서 무료로 사용하는 방법은 무엇입니까? 위의 API를 사용하여 개발된 다음 최고의 무료 PDF-JPG 변환기 온라인 고품질을 사용해 보십시오.
결론
이 문서에서는 다음을 배웠습니다.
- 클라우드의 Node.js에서 PDF를 JPG 형식으로 변경하는 방법
- 프로그래밍 방식으로 PDF 파일을 업로드한 다음 클라우드에서 변환된 파일을 다운로드합니다.
- 클라우드 스토리지를 사용하지 않고 PDF를 온라인에서 무료 고품질로 jpg로 변환;
- 고급 옵션을 사용하여 Node.js에서 pdf를 jpg로 변환하는 방법
- Node.js를 사용하여 변환된 PDF 문서에 워터마크를 추가하는 방법
문서를 사용하여 GroupDocs.Conversion Cloud API에 대해 자세히 알아볼 수 있습니다. 또한 브라우저를 통해 직접 API를 시각화하고 상호 작용할 수 있는 API 참조 섹션을 제공합니다. 또한 시작하기 가이드를 참조하는 것이 좋습니다.
또한 blog.groupdocs.cloud는 흥미로운 주제에 대한 새로운 블로그 게시물을 작성하고 있습니다. 최신 정보는 문의해 주십시오.
질문하기
무료 지원 포럼을 사용하여 PDF를 JPG 형식으로 변환하는 방법에 대한 질문을 할 수 있습니다.
FAQ
Node.js에서 PDF를 JPG로 어떻게 변환합니까?
PDF를 JPG 파일로 빠르고 쉽게 변환하는 방법에 대한 Node.js 코드 스니펫을 배우려면 이 링크를 따르십시오.
REST API를 사용하여 PDF를 JPG 파일로 변환하는 방법은 무엇입니까?
ConvertApi 인스턴스를 만들고, 변환 설정 값을 설정하고, PDF를 JPG 파일로 변환하기 위해 ConvertDocumentRequest와 함께 convertDocument 메서드를 호출합니다.
온라인에서 무료로 PDF를 JPG로 변환하는 방법은 무엇입니까?
온라인 무료 PDF-JPG 변환기를 사용하면 PDF를 JPG 형식으로 빠르고 쉽게 내보낼 수 있습니다. 변환이 완료되면 JPG 파일을 다운로드할 수 있습니다.
온라인에서 무료로 PDF 파일을 JPG로 변환하는 방법은 무엇입니까?
- 온라인 PDF-JPG 변환기 무료 열기
- 파일 드롭 영역 내부를 클릭하여 PDF를 업로드하거나 PDF 파일을 끌어다 놓습니다.
- 지금 변환 버튼을 클릭하면 온라인 PDF-JPG 변환기 소프트웨어가 PDF 파일을 JPG로 변환합니다.
- 출력 파일의 다운로드 링크는 PDF를 JPG 파일로 변환한 후 즉시 사용할 수 있습니다.
PDF to JPG 형식 변환기 무료 다운로드 라이브러리를 설치하는 방법은 무엇입니까?
PDF를 JPG 변환기로 무료로 설치Node.js 라이브러리 다운로드를 통해 프로그래밍 방식으로 PDF를 생성하고 JPG로 변환하세요.
Windows에서 오프라인으로 PDF를 JPG로 어떻게 변환합니까?
Windows용 무료 PDF-JPG 변환기 소프트웨어를 다운로드하려면 이 링크를 방문하십시오. 이 온라인 PDF to JPG 변환기 무료 다운로드 소프트웨어를 사용하면 클릭 한 번으로 Windows에서 PDF를 JPG로 빠르게 변환할 수 있습니다.
또한보십시오
자세한 내용은 다음 문서를 참조하십시오.
- Python에서 REST API를 사용하여 Word 문서를 PDF로 변환
- Python SDK를 사용하여 PDF를 편집 가능한 Word 문서로 변환
- Ruby에서 REST API를 사용하여 온라인에서 PowerPoint PPT/PPTX 파일 병합
- Python에서 MSG 및 EML 파일을 PDF로 변환
- Python에서 XML을 CSV로, CSV를 XML로 변환
- Python에서 CSV를 JSON으로, JSON을 CSV로 변환하는 방법
- Java에서 CSV를 JSON으로, JSON을 CSV로 변환
- Python에서 EXCEL을 JSON으로, JSON을 EXCEL로 변환하는 방법
- Python에서 마크다운을 PDF로, PDF를 마크다운으로 변환
- Python에서 Word를 Markdown으로, Markdown을 Word로 변환
- REST API를 사용하여 C#에서 HTML을 PDF로 변환하는 방법
- C#에서 프로그래밍 방식으로 Word를 PDF로 변환하는 방법