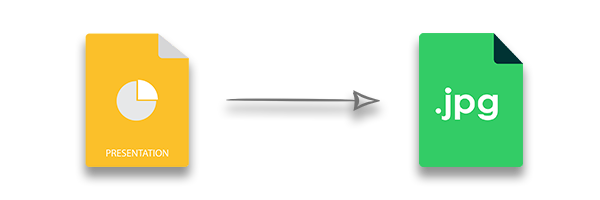
Node.js에서 PowerPoint를 JPG로, JPG를 PowerPoint로 변환
PowerPoint PPT 또는 PPTX를 클라우드에서 프로그래밍 방식으로 JPG/JPEG와 같은 널리 사용되는 형식의 이미지로 변환합니다. Node.js 개발자는 Node.js 애플리케이션에서 온라인으로 PPT 또는 PPTX를 고해상도 JPG로 쉽게 변환할 수 있습니다. 이 기사는 Node.js에서 PowerPoint를 JPG로 변환하고 JPG를 PowerPoint로 변환하는 방법에 중점을 둘 것입니다.
이 문서에서는 다음 항목을 다룹니다.
- Node.js 이미지를 PPT 변환기로 변환하고 PPT 슬라이드를 이미지 API로 변환 - 설치
- Node.js에서 REST API를 사용하여 온라인에서 PPTX를 JPG로 변환하는 방법
- 고급 옵션을 사용하여 PowerPoint 슬라이드를 Node.js에서 JPG로 변환
- Node.js에서 JPG를 PowerPoint 프레젠테이션으로 변환하는 방법
- 고급 옵션을 사용하여 Node.js에서 JPG를 PPTX PowerPoint 슬라이드로 변환
Node.js 이미지를 PPT로 변환 및 PPT 슬라이드를 이미지 API로 변환 - 설치
PowerPoint PPTX를 JPG로 변환하기 위해 GroupDocs.Conversion Cloud의 Node.js SDK API를 사용할 것입니다. API를 사용하면 문서를 필요한 형식으로 변환할 수 있습니다. Word, Excel, PowerPoint, PDF, HTML, JPG, PNG, CAD와 같은 50개 이상의 문서 및 이미지 변환을 지원합니다. 또한 Cloud API용 문서 변환 제품군으로 .NET, Java, PHP, Ruby, Android 및 Python SDK를 제공합니다.
콘솔에서 다음 명령을 사용하여 GroupDocs.Conversion Cloud를 Node.js 애플리케이션에 설치할 수 있습니다.
npm install groupdocs-conversion-cloud
단계와 사용 가능한 코드 예제를 시작하기 전에 대시보드에서 클라이언트 ID와 클라이언트 암호를 얻으십시오. ID와 시크릿이 있으면 아래와 같이 코드를 추가합니다.
# http://api.groupdocs.cloud에서 노드 애플리케이션의 Node.js SDK 가져오기
global.groupdocs_conversion_cloud = require("groupdocs-conversion-cloud");
global.fs = require("fs");
// https://dashboard.groupdocs.cloud에서 clientId 및 clientSecret을 가져옵니다(무료 등록 필요).
global.clientId = "xxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx";
global.clientSecret = "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx";
global.myStorage = "test-internal-storage";
const config = new groupdocs_conversion_cloud.Configuration(clientId, clientSecret);
config.apiBaseUrl = "https://api.groupdocs.cloud";
Node.js에서 REST API를 사용하여 온라인에서 PPTX를 JPG로 변환하는 방법
아래의 간단한 단계에 따라 PowerPoint PPT 또는 PPTX를 JPG 이미지로 변환할 수 있습니다.
파일 업로드
먼저 다음 코드 샘플을 사용하여 PowerPoint 파일을 클라우드에 업로드합니다.
// 시스템 드라이브에서 IOStream의 파일을 엽니다.
var resourcesFolder = 'H:\\groupdocs-cloud-data\\sample-pptx-file';
// 파일 읽기
fs.readFile(resourcesFolder, (err, fileStream) => {
// FileApi 구성
var fileApi = groupdocs_conversion_cloud.FileApi.fromConfig(config);
// 업로드 파일 요청 생성
var request = new groupdocs_conversion_cloud.UploadFileRequest("sample-pptx-file", fileStream, myStorage);
// 파일 업로드
fileApi.uploadFile(request)
.then(function (response) {
console.log("Expected response type is FilesUploadResult: " + response.uploaded.length);
})
.catch(function (error) {
console.log("Error: " + error.message);
});
});
결과적으로 업로드된 PowerPoint 파일은 클라우드 대시보드의 파일 섹션에서 사용할 수 있습니다.
Node.js에서 PowerPoint를 JPG 고해상도로 변환
프로그래밍 방식으로 PowerPoint PPTX를 JPG 파일로 변환하려면 아래에 언급된 단계를 따르십시오.
- ConvertApi의 인스턴스 생성
- ConvertSettings 인스턴스 만들기
- 이제 클라우드 스토리지 이름을 설정하십시오.
- PowerPoint PPTX 파일 경로 설정
- 이제 형식에 “jpg"를 할당합니다.
- 다음으로 출력 파일 경로를 제공하십시오.
- ConvertDocumentRequest 인스턴스 만들기
- 마지막으로 ConvertApi.convertDocument() 메서드를 호출하여 PPTX를 JPG 형식으로 변환합니다.
다음 코드 예제는 Node.js에서 REST API를 사용하여 PowerPoint를 JPG 형식으로 변환하는 방법을 보여줍니다.
// Node.js에서 REST API를 사용하여 온라인에서 PPTX를 JPG로 변환하는 방법
const convert = async () => {
const convertApi = groupdocs_conversion_cloud.ConvertApi.fromKeys(clientId, clientSecret);
const settings = new groupdocs_conversion_cloud.ConvertSettings();
settings.storageName = myStorage;
settings.filePath = "nodejs-testing/sample-pptx-file.pptx";
settings.format = "jpg";
settings.outputPath = "nodejs-testing/output-sample-file.jpg";
try {
// 변환 문서 요청 생성
const request = new groupdocs_conversion_cloud.ConvertDocumentRequest(settings);
await convertApi.convertDocument(request);
}
catch (err) {
throw err;
}
}
convert()
.then(() => {
console.log("Successfully converted PowerPoint to JPG file format.");
})
.catch((err) => {
console.log("Error occurred while converting the PowerPoint file:", err);
})
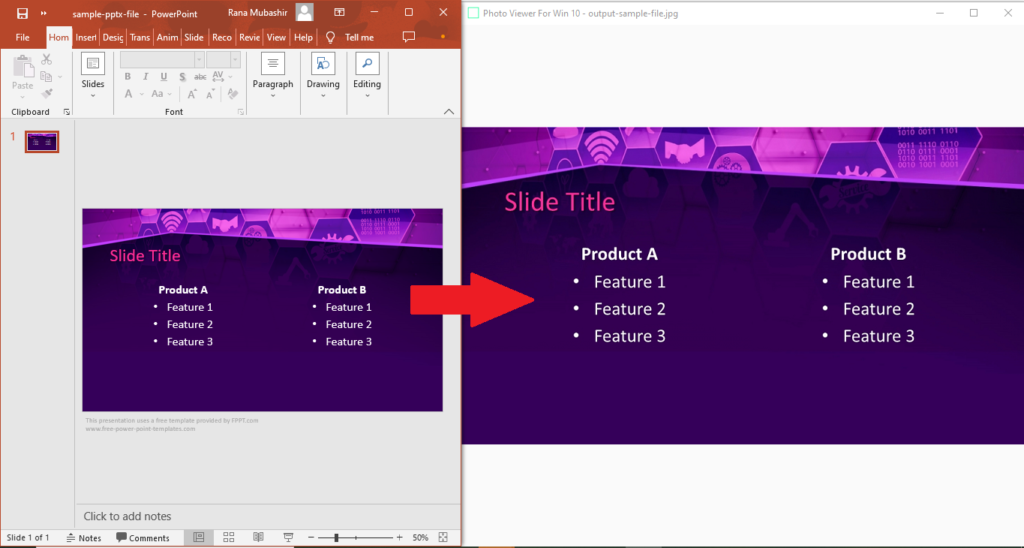
Node.js에서 PowerPoint를 JPG 고해상도로 변환
변환된 파일 다운로드
위의 코드 샘플은 변환된 JPG 파일을 클라우드에 저장합니다. 다음 코드 샘플을 사용하여 다운로드할 수 있습니다.
// FileApi를 구성하여 변환된 파일 다운로드
var fileApi = groupdocs_conversion_cloud.FileApi.fromConfig(config);
// 다운로드 파일 요청 생성
let request = new groupdocs_conversion_cloud.DownloadFileRequest("nodejs-testing/output-sample-file.jpg", myStorage);
// 다운로드 파일 및 응답 유형 스트림
fileApi.downloadFile(request)
.then(function (response) {
// 시스템 디렉토리에 파일 저장
fs.writeFile("H:\\groupdocs-cloud-data\\output-sample-file.jpg", response, "binary", function (err) { });
console.log("Expected response type is Stream: " + response.length);
})
.catch(function (error) {
console.log("Error: " + error.message);
});
고급 옵션을 사용하여 Node.js에서 PowerPoint 슬라이드를 JPG로 변환
몇 가지 고급 설정을 사용하여 온라인에서 PPTX를 고품질 JPG로 변환하려면 아래에 언급된 단계를 따르십시오.
- ConvertApi의 인스턴스 생성
- ConvertSettings 인스턴스 만들기
- 이제 클라우드 스토리지 이름을 설정하십시오.
- 이제 PPTX 입력 파일 경로를 설정하십시오.
- 다음으로 형식에 “jpg"를 지정합니다.
- JpgConvertOptions 인스턴스 정의
- grayscale, fromPage, pagesCount, quality, rotateAngle, usePdf 등과 같은 다양한 변환 설정을 지정합니다.
- convertOptions 및 결과 출력 파일 경로 설정
- ConvertDocumentRequest 인스턴스 만들기
- 마지막으로 ConvertApi.convertDocument() 클래스를 호출하여 PPTX를 JPG 이미지로 변환합니다.
다음 코드 예제는 고급 변환 설정을 사용하여 온라인에서 PPTX를 JPG 형식으로 변환하는 방법을 보여줍니다.
// 고급 옵션을 사용하여 Node.js에서 PowerPoint 슬라이드를 JPG로 변환하는 방법
const convert_options = async () => {
const convertApi = groupdocs_conversion_cloud.ConvertApi.fromKeys(clientId, clientSecret);
const settings = new groupdocs_conversion_cloud.ConvertSettings();
settings.storageName = myStorage;
settings.filePath = "nodejs-testing/sample-pptx-file.pptx";
settings.format = "jpg";
convertOptions = new groupdocs_conversion_cloud.JpgConvertOptions()
convertOptions.grayscale = true;
convertOptions.fromPage = 1;
convertOptions.pagesCount = 1;
convertOptions.quality = 100;
convertOptions.rotateAngle = 90;
convertOptions.usePdf = false;
settings.convertOptions = convertOptions;
settings.outputPath = "nodejs-testing/output-sample-file.jpg";
try {
// 변환 문서 요청 생성
const request = new groupdocs_conversion_cloud.ConvertDocumentRequest(settings);
await convertApi.convertDocument(request);
}
catch (err) {
throw err;
}
}
convert_options()
.then(() => {
console.log("Converted PPT to JPG image with advanced options.");
})
.catch((err) => {
console.log("Error occurred while converting the PPT file:", err);
})
Node.js에서 JPG를 PowerPoint 프레젠테이션으로 변환하는 방법
프로그래밍 방식으로 JPG 파일을 PPTX로 변환하려면 아래에 언급된 단계를 따르십시오.
- ConvertApi의 인스턴스 생성
- ConvertSettings 인스턴스 만들기
- 이제 클라우드 스토리지 이름을 설정하십시오.
- 다음으로 입력 JPG 파일 경로를 설정하십시오.
- 출력 형식을 “pptx"로 설정
- 다음으로 출력 파일 경로를 제공하십시오.
- ConvertDocumentRequest 메서드를 사용하여 개체 만들기
- 마지막으로 ConvertApi.convertDocument() 메서드를 호출하여 결과를 얻습니다.
다음 코드 예제는 Node.js에서 REST API를 사용하여 JPG 파일을 PowerPoint 형식으로 변환하는 방법을 보여줍니다.
// Node.js에서 JPG 파일을 PowerPoint 프레젠테이션으로 변환하는 방법
const convert = async () => {
const convertApi = groupdocs_conversion_cloud.ConvertApi.fromKeys(clientId, clientSecret);
const settings = new groupdocs_conversion_cloud.ConvertSettings();
settings.storageName = myStorage;
settings.filePath = "nodejs-testing/sample-jpg-file.jpg";
settings.format = "pptx";
settings.outputPath = "nodejs-testing/output-sample-file.pptx";
try {
// 변환 문서 요청 생성
const request = new groupdocs_conversion_cloud.ConvertDocumentRequest(settings);
await convertApi.convertDocument(request);
}
catch (err) {
throw err;
}
}
//
convert()
.then(() => {
console.log("Successfully converted JPG to PPTX file format.");
})
.catch((err) => {
console.log("Error occurred while converting the JPG file:", err);
})
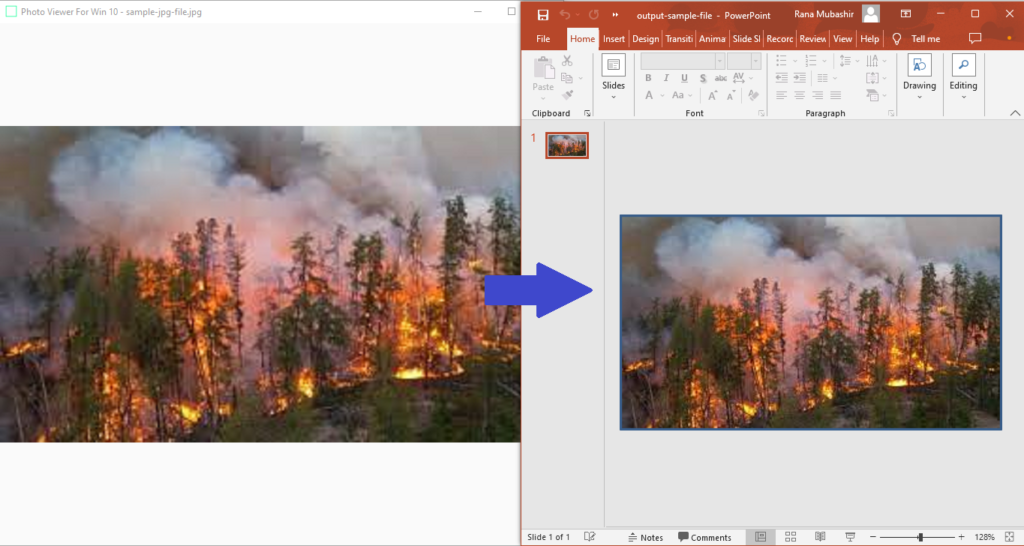
Node.js에서 JPG를 PowerPoint 슬라이드로 변환
고급 옵션을 사용하여 Node.js에서 JPG를 PPTX PowerPoint 슬라이드로 변환
몇 가지 고급 설정을 사용하여 JPG 이미지를 PPXT 온라인 변환기 API로 사용하여 아래 언급된 단계를 따르십시오.
- ConvertApi의 인스턴스 생성
- ConvertSettings 인스턴스 만들기
- 이제 클라우드 스토리지 이름을 설정하십시오.
- 다음으로 JPG 파일 경로를 설정합니다.
- 이제 형식에 “pptx"를 지정하십시오.
- PptxConvertOptions 정의
- fromPage, pagesCount, zoom 등과 같은 다양한 변환 설정을 지정합니다.
- convertOptions 및 결과 출력 파일 경로 설정
- ConvertDocumentRequest 생성 및 인스턴스
- 이제 ConvertApi.convertDocument() 메서드를 호출하여 결과를 얻습니다.
다음 코드 예제는 고급 변환 옵션을 사용하여 온라인에서 JPG 형식을 PPTX로 변환하는 방법을 보여줍니다.
// 고급 옵션을 사용하여 Node.js에서 JPG 파일을 PowerPoint PPTX로 변환하는 방법
const convert_options = async () => {
const convertApi = groupdocs_conversion_cloud.ConvertApi.fromKeys(clientId, clientSecret);
const settings = new groupdocs_conversion_cloud.ConvertSettings();
settings.storageName = myStorage;
settings.filePath = "nodejs-testing/sample-jpg-file.jpg";
settings.format = "pptx";
var convertOptions = new groupdocs_conversion_cloud.PptxConvertOptions();
convertOptions.fromPage = 1;
convertOptions.pagesCount = 1;
convertOptions.zoom = 1;
settings.convertOptions = convertOptions;
settings.outputPath = "nodejs-testing/output-sample-file.pptx";
try {
// 변환 문서 요청 생성
const request = new groupdocs_conversion_cloud.ConvertDocumentRequest(settings);
await convertApi.convertDocument(request);
}
catch (err) {
throw err;
}
}
convert_options()
.then(() => {
console.log("Converted JPG file into PPTX file using advanced options.");
})
.catch((err) => {
console.log("Error occurred while converting the JPG file:", err);
})
JPG 변환기 무료 온라인 파워포인트
온라인 무료 PPT to JPG 변환기는 무엇입니까? 위의 API를 사용하여 개발된 다음 PPT에서 이미지 변환기 온라인을 사용해 온라인에서 PPT를 JPG로 변환하십시오.
온라인에서 무료 JPG를 PPT로 변환
온라인에서 무료로 JPG를 PPT로 변환하는 방법은 무엇입니까? 위의 API를 사용하여 개발된 다음 온라인 JPG에서 PPT 변환기를 사용하여 JPG를 무료로 편집 가능한 PPT로 변환하십시오.
합산
이번 블로그 포스팅은 여기서 마치겠습니다. 이 문서에서는 다음 내용을 다뤘습니다.
- 클라우드에서 PowerPoint PPTX를 JPG 형식으로 변환하는 방법
- 일부 고급 옵션을 사용하여 PPTX를 JPG로 변환하는 방법
- PPTX 파일을 업로드한 다음 클라우드에서 변환된 JPG 파일을 다운로드합니다.
- 클라우드에서 JPG 형식을 PowerPoint 파일로 변환하는 방법
- 고급 옵션을 사용하여 Node.js에서 JPG 파일을 PPTX 파일로 변환하는 방법
또한 브라우저를 통해 직접 API를 시각화하고 상호 작용할 수 있는 API 참조 섹션을 제공합니다. 또한 시작 가이드를 따라 고급 변환 기능을 사용하는 것이 좋습니다!.
마지막으로 blog.groupdocs.cloud는 새롭고 흥미로운 블로그 기사를 작성하고 있습니다. 따라서 정기 업데이트와 최신 업데이트를 계속 확인하십시오.
질문하기
포럼을 통해 PowerPoint PPTX를 JPG 형식으로 또는 그 반대로 변환하는 방법에 대한 질문을 자유롭게 하십시오.
FAQ
Node.js에서 PowerPoint를 JPG로 어떻게 변환합니까?
PowerPoint를 JPG 파일로 빠르고 쉽게 변환하는 방법에 대한 Node.js 코드 스니펫을 배우려면 이 링크를 따르십시오.
REST API를 사용하여 PowerPoint를 JPG 파일로 변환하는 방법은 무엇입니까?
ConvertApi의 인스턴스를 만들고 변환 설정 값을 설정하고 PowerPoint를 JPG 파일로 변환하기 위해 ConvertDocumentRequest와 함께 convertDocument 메서드를 호출합니다.
온라인에서 무료로 PowerPoint를 JPG로 변환하는 방법은 무엇입니까?
온라인 무료 PowerPoint-JPG 변환기를 사용하면 PowerPoint를 빠르고 쉽게 JPG 형식으로 내보낼 수 있습니다. 변환이 완료되면 JPG 파일을 다운로드할 수 있습니다.
온라인에서 무료로 PowerPoint 파일을 JPG로 변환하는 방법은 무엇입니까?
- 온라인 PowerPoint에서 JPG 변환기 무료 열기
- 파일 드롭 영역 내부를 클릭하여 PowerPoint를 업로드하거나 PowerPoint 파일을 끌어다 놓습니다.
- 지금 변환 버튼을 클릭하면 온라인 PowerPoint에서 JPG로 변환 소프트웨어가 PowerPoint 파일을 JPG로 변환합니다.
- 출력 파일의 다운로드 링크는 PowerPoint를 JPG 파일로 변환한 후 즉시 사용할 수 있습니다.
JPG 형식 변환기 무료 다운로드 라이브러리에 PowerPoint를 설치하는 방법은 무엇입니까?
PowerPoint to JPG 변환기 무료 다운로드Node.js 라이브러리를 설치하여 프로그래밍 방식으로 PowerPoint를 생성하고 JPG로 변환합니다.
Windows에서 오프라인으로 PowerPoint를 JPG로 어떻게 변환합니까?
Windows용 PowerPoint를 JPG로 변환하는 소프트웨어를 무료로 다운로드하려면 이 링크를 방문하세요. 이 온라인 PowerPoint to JPG 변환기 무료 다운로드 소프트웨어를 사용하면 클릭 한 번으로 Windows에서 PowerPoint를 JPG로 빠르게 전환할 수 있습니다.
Node.js에서 JPG를 PPT 파일로 어떻게 변환합니까?
JPG를 PPT 파일로 빠르고 쉽게 변환하는 방법에 대한 Node.js 코드 스니펫을 배우려면 이 링크를 따르십시오.
REST API를 사용하여 JPG 이미지를 PowerPoint 파일로 변환하는 방법은 무엇입니까?
ConvertApi의 인스턴스를 만들고 변환 설정 값을 설정하고 JPG 파일을 PowerPoint 파일로 변환하기 위해 ConvertDocumentRequest와 함께 convertDocument 메서드를 호출합니다.
온라인에서 무료로 JPG를 PPTX로 변환하는 방법?
온라인 JPG에서 PPTX로 무료 변환기를 사용하면 JPG를 PPTX 파일 형식으로 쉽고 빠르게 가져올 수 있습니다. 변환이 완료되면 PPTX 파일을 다운로드할 수 있습니다.
온라인에서 무료로 JPG 파일을 PPTX로 변환하는 방법은 무엇입니까?
- 온라인 JPG에서 PPTX로 무료 변환기 열기
- 파일 드롭 영역 내부를 클릭하여 JPG를 업로드하거나 JPG 파일을 끌어다 놓습니다.
- 지금 변환 버튼을 클릭하면 온라인 JPG에서 PPTX로 변환 소프트웨어가 JPG 파일을 PPTX로 변환합니다.
- 출력 파일의 다운로드 링크는 JPG를 PPTX 파일로 변환한 후 즉시 사용할 수 있습니다.
JPG to PowerPoint 형식 변환기 무료 다운로드 라이브러리를 설치하는 방법은 무엇입니까?
JPG를 PowerPoint 변환기로 설치Node.js 라이브러리 무료 다운로드를 설치하고 프로그래밍 방식으로 JPG를 PowerPoint로 변환합니다.
Windows에서 오프라인으로 JPG를 PPT로 어떻게 변환합니까?
Windows용 무료 JPG-PPT 변환기 소프트웨어를 다운로드하려면 이 링크를 방문하십시오. 이 온라인 JPG to PPT 변환기 무료 다운로드 소프트웨어를 사용하면 클릭 한 번으로 Windows에서 JPG를 PPTX로 빠르게 전환할 수 있습니다.
또한보십시오
다음 관련 문서를 방문하여 자세히 알아보는 것이 좋습니다.
- Python에서 REST API를 사용하여 Word 문서를 PDF로 변환
- Python SDK를 사용하여 PDF를 편집 가능한 Word 문서로 변환
- Ruby에서 REST API를 사용하여 온라인에서 PowerPoint PPT/PPTX 파일 병합
- Python에서 MSG 및 EML 파일을 PDF로 변환
- Python에서 XML을 CSV로, CSV를 XML로 변환
- Python에서 CSV를 JSON으로, JSON을 CSV로 변환하는 방법
- Java에서 CSV를 JSON으로, JSON을 CSV로 변환
- Python에서 EXCEL을 JSON으로, JSON을 EXCEL로 변환하는 방법
- Python에서 마크다운을 PDF로, PDF를 마크다운으로 변환
- Python에서 Word를 Markdown으로, Markdown을 Word로 변환
- REST API를 사용하여 C#에서 HTML을 PDF로 변환하는 방법
- C#에서 프로그래밍 방식으로 Word를 PDF로 변환하는 방법
- Java에서 프로그래밍 방식으로 Word를 JPG로, JPG를 Word로 변환