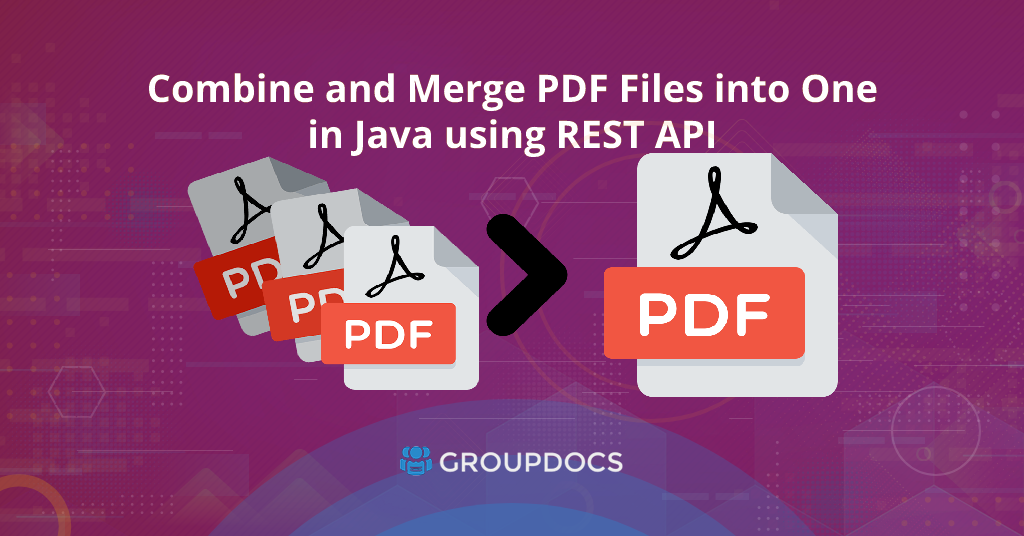
Combine and Merge PDF Files into One in Java using REST API.
Merging PDF documents provides a simple and effective way to manage multiple files, saving storage space, streamlining workflow, and making it easy to share files on any platform. The GroupDocs.Merger Cloud SDK for Java provides an efficient and straightforward solution to this problem. You can quickly combine PDF files programmatically in Java, and save valuable time and effort. In this article, we’ll demonstrate how to combine and merge PDF files into one in Java using REST API.
The following topics shall be covered in this article:
Java PDF Files Merger REST API and SDK Installation
GroupDocs.Merger Cloud SDK for Java is a powerful document manipulation tool that allows developers to combine, split, rotate, change the page orientation as portrait or landscape, and modify documents in the cloud. It is a cloud-based document manipulation and cross-platform API that supports various file formats, including Word documents, PDFs, Excel spreadsheets, PowerPoint presentations, HTML, and more. The SDK is easy to use and can be integrated into a Java-based application.
You can either download the API’s JAR file or install it using Maven by adding the following repository and dependency into your project’s pom.xml file:
Maven Repository:
<repository>
<id>groupdocs-artifact-repository</id>
<name>GroupDocs Artifact Repository</name>
<url>https://repository.groupdocs.cloud/repo</url>
</repository>
Maven Dependency:
<dependency>
<groupId>com.groupdocs</groupId>
<artifactId>groupdocs-merger-cloud</artifactId>
<version>23.2</version>
<scope>compile</scope>
</dependency>
Now, sign up for a free trial account or purchase a subscription plan on the GroupDocs website and get your API key. Once you have the Client Id and Client Secret, add below code snippet to a Java-based application:
How to Merge Two PDF Files into One Using Java
To merge PDF files using GroupDocs.Merger Cloud SDK for Java, you will need to follow below simple steps:
- Upload the PDF files to the cloud
- Combine multiple PDF documents into one in Java
- Download the merged PDF documents
Upload the Files
Firstly, upload the PDF files to the cloud using the code example given below:
As a result, the uploaded PDF files will be available in the files section of your dashboard on the cloud.
Combine PDF Pages into One File
This section provides a step-by-step guide and an example code snippet on how to merge all PDF documents into one:
- Firstly, import the required classes into your Java file.
- Secondly, create an instance of the DocumentApi class.
- Thirdly, create an instance of the FileInfo class.
- Next, call the setFilePath() method and pass the input file path as a parameter.
- Then, create an instance of the JoinItem class.
- Now, call the setFileInfo() method and pass the fileInfo1 parameter.
- Next, create a second instance of the FileInfo and JoinItem classes.
- Provide the input file path and fileInfo2 parameters.
- Add more JoinItems for merging more than two documents.
- After that, create an instance of the JoinOptions() class.
- Then, add a comma-separated list of created join items.
- Next, set the output file path.
- Now, create an instance of the JoinRequest() class and pass the JoinOptions parameter.
- Finally, merge PDF files by calling the join() method of the DocumentApi and passing the JoinRequest parameter.
The following code snippet shows how to merge multiple PDF files into one file in Java using REST API:
Download the File
The above code sample will save the merged PDF file on the cloud. You can download it using the following code sample:
Free Online PDF Files Merger
How to merge PDF files online for free? Please try the free PDF Merger to combine multiple PDF files into one online. This online document merger is developed using the above-mentioned Groupdocs.Merger Cloud APIs.
Conclusion
The GroupDocs.Merger Cloud SDK for Java is the ideal solution for the quick and easy merging of PDF documents, freeing up your time and effort. The following is what you have learned from this article:
- how to combine and merge multiple PDF files on the cloud using Java;
- programmatically upload and download the merged PDF file in Java;
- and merge PDF files online for free using a free online PDF document merger.
Moreover, we also provide an API Reference section that lets you visualize and communicate with our APIs directly through the browser. Java SDK’s complete source code is freely available on Github. Please check the GroupDocs.Merger Cloud SDK for Java Examples here.
Additionally, we suggest you follow our Getting Started guide for detailed steps and API usage.
Finally, we keep writing new blog posts on different document operations using REST API. So, please get in touch for the latest updates.
Ask a question
If you have any questions about the PDF Files Merger API, please feel free to ask us on the Free Support Forum.
FAQs
How do I merge multiple PDF files into one in Java?
You can combine and merge multiple PDF files into one using GroupDocs.Merger Cloud SDK for Java.
Can I merge specific pages from multiple PDF files in Java?
Yes, you can use GroupDocs.Merger Cloud SDK for Java to merge specific pages from multiple PDF documents.
Is GroupDocs.Merger Cloud SDK for Java a secure platform for merging PDF files?
Yes, GroupDocs.Merger Cloud SDK for Java is a secure solution for merging PDF documents, providing encryption, and other security features to ensure the safety of your data.
Can I combine other file formats using GroupDocs.Merger Cloud SDK for Java?
Yes, GroupDocs.Merger Cloud SDK for Java supports merging documents of various other formats, including PDF, PowerPoint, HTML, Word, and more.
See Also
For further information on related topics, we suggest taking a look at the following articles:
- Split Word Documents into Separate Files in Java
- How to Split PowerPoint PPT or PPTX Slides in Python
- Extract Specific Pages from PDF using Python
- Extract Pages From Word Documents using Rest API
- Merge PowerPoint PPT/PPTX Files Online using REST API
- How to Rotate PDF Pages using Rest API in Ruby
- How to Change Page Orientation in Word Document using Ruby
- Merge and Combine PDF Files using REST API in Ruby
- Extract Pages from PDF in Java - Separate PDF Pages Online