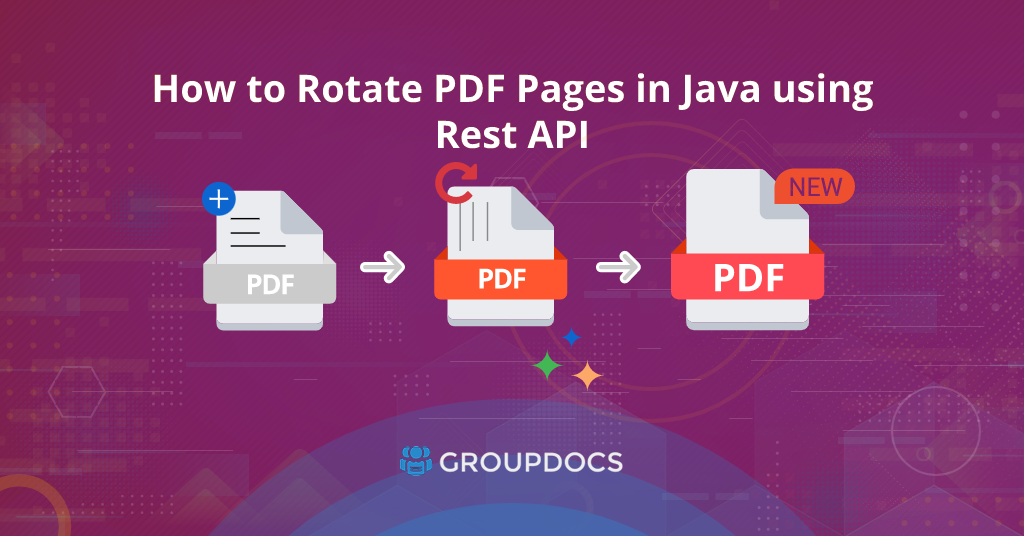
How to Rotate PDF Pages in Java using Rest API.
PDF files are widely used for various purposes, including document sharing, archiving, and printing. However, there are situations where you may need to rotate all or specific pages in a PDF file programmatically. Whether you want to correct the orientation of scanned pages or adjust the layout for better readability, rotating PDF pages is a common requirement. In this article, we will explore how to rotate PDF pages in Java using REST API.
The following topics shall be covered in this article:
- Java REST API to Rotate PDF Pages Online and SDK Installation
- How to Rotate All Pages in PDF File using Java
- How to Rotate Specific Pages of PDF File in Java
Java REST API to Rotate PDF Pages Online and SDK Installation
GroupDocs.Merger Cloud SDK for Java is a powerful and reliable solution that allows you to manipulate PDF documents programmatically. It provides a wide range of features that make it easy to split, merge, reorder, rotate, swap, and manipulate PDF documents. The SDK is easy to use and can be integrated into a Java-based application to automate file manipulation tasks.
You can either download the API’s JAR file or install it using Maven by adding the following repository and dependency into your project’s pom.xml file:
Maven Repository:
<repository>
<id>groupdocs-artifact-repository</id>
<name>GroupDocs Artifact Repository</name>
<url>https://repository.groupdocs.cloud/repo</url>
</repository>
Maven Dependency:
<dependency>
<groupId>com.groupdocs</groupId>
<artifactId>groupdocs-merger-cloud</artifactId>
<version>23.2</version>
<scope>compile</scope>
</dependency>
Now, you need to sign up for a free trial account or purchase a subscription plan on the GroupDocs website to get your API key. Once you have the Client Id and Client Secret, add below code snippet to a Java-based application:
How to Rotate All Pages in PDF File using Java
Rotating PDF file pages with GroupDocs.Merger Cloud SDK is a straightforward process. Follow these steps to rotate all pages within a PDF file:
Upload the Files
Firstly, upload the PDF file to the cloud using the code example given below:
As a result, the uploaded PDF file will be available in the files section of your dashboard on the cloud.
Rotate PDF File Pages in Java
By following the steps and an example code snippet, you can easily rotate PDF pages programmatically using GroupDocs.Merger Cloud SDK in your Java application:
- Firstly, import the required classes into your Java file.
- Secondly, create an instance of the PagesApi class.
- Thirdly, create an instance of the FileInfo class.
- Now, provide the input PDF document path.
- Then, create an instance of the RotateOptions class.
- Now, set the fileInfo and sample output file path.
- Next, set the desired page rotation like Rotate90, Rotate180, or Rotate270.
- After that, create the RotateRequest class instance and pass the RotateOptions parameter.
- Finally, call the rotate() method and pass the RotateRequest parameter to rotate PDF file pages.
The following code snippet shows how to rotate all pages of a PDF file in Java using REST API:
Download the File
The above code sample will save the rotated PDF file on the cloud. You can download it using the following code sample:
How to Rotate Specific Pages of PDF File in Java
If you want to rotate only specific pages of a PDF file, the SDK allows you to define the page range accordingly. Here are the steps and an example code snippet to set custom rotation angles:
- Firstly, import the required classes into your Java file.
- Secondly, create an instance of the PagesApi class.
- Thirdly, create an instance of the FileInfo class.
- Now, provide the input PDF document path.
- Then, create an instance of the RotateOptions class.
- Now, set the fileInfo and sample output file path.
- Set the desired page numbers in the page collection array.
- Next, set the desired page rotation like Rotate90, Rotate180, or Rotate270.
- After that, create the RotateRequest class instance and pass the RotateOptions parameter.
- Finally, call the rotate() method and pass the RotateRequest parameter to rotate PDF file pages.
The following code snippet elaborates on how to rotate specific or certain pages in a PDF document using Java:
Rotate PDF Pages Free Online
How to rotate PDF pages online for free? Please try the following free online tool to rotate PDF file pages. This tool is developed using the above-mentioned Groupdocs.Merger Cloud APIs.
Conclusion
With GroupDocs.Merger Cloud SDK for Java, you can effortlessly rotate PDF pages, enhance document readability, and improve the user experience. The following is what you have learned in this article:
- how to rotate all pages in a PDF document using Java;
- programmatically upload and download the files in Java on the cloud;
- how to rotate specific pages of PDF files using Java;
- and rotate PDF file pages for free using an online PDF rotation tool.
Additionally, we also provide an API Reference section that lets you visualize and communicate with our APIs directly through the browser. Java SDK’s complete source code is freely available on Github. Please check the GroupDocs.Merger Cloud SDK for Java Examples here.
Moreover, we suggest you follow our Getting Started guide for detailed steps and API usage.
Finally, we keep writing new blog posts on different document operations using REST API. So, please get in touch for the regular updates.
Ask a question
If you have any questions about the PDF Pages Rotation API, please feel free to ask us on the Free Support Forum.
FAQs
Can I rotate specific pages within a PDF document using GroupDocs.Merger Cloud SDK for Java?
Yes, you can specify the page range to rotate specific pages within a PDF document using GroupDocs.Merger Cloud SDK for Java.
How do I rotate PDF pages online in Java?
Create an instance of PagesApi, set the values of the RotateOptions, and invoke the pagesApi.rotate() method with RotateRequest to rotate PDF pages and save them online using Java.
How do I rotate PDF file pages on Windows?
Please visit this link to download the PDF pages rotation tool. This offline software is used to perform different file format operations, including document rotation in Windows.
See Also
Here are some related articles that you may find helpful:
- Merge Multiple JPG Files into One in Java | Merge JPG to JPG
- Combine and Merge PDF Files into One in Java using REST API
- How to Split PowerPoint PPT or PPTX Slides in Python
- Merge PowerPoint PPT/PPTX Files Online using REST API
- How to Change Page Orientation in Word Document using Ruby
- How to Split Word Documents into Separate Files using Node.js
- Extract Pages from PDF in Java - Separate PDF Pages Online
- Extract Document Pages - Extract Pages from Word File in Java