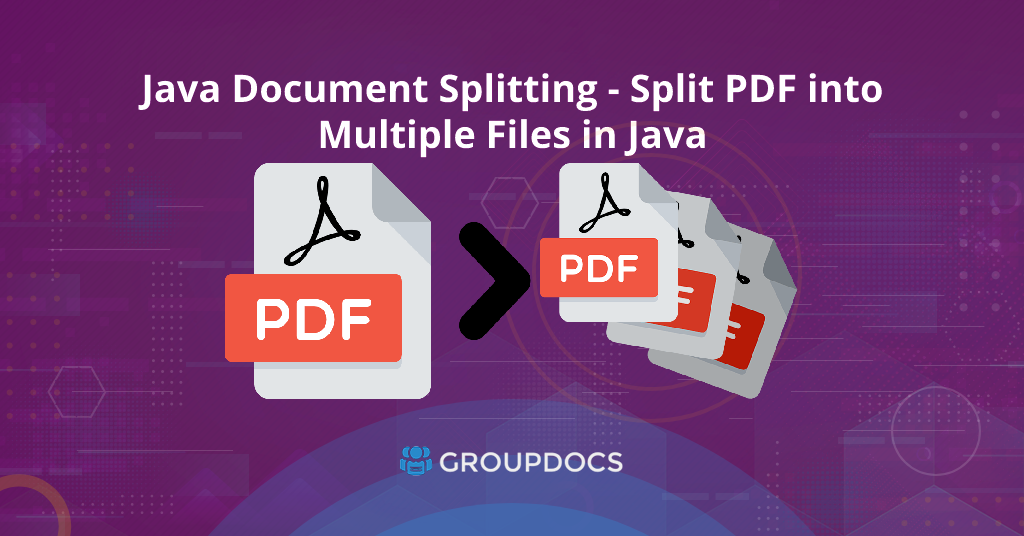
Java Document Splitting - Split PDF into Multiple Files in Java.
Are you looking for an easy and efficient way to split PDF documents into separate files in your Java applications? With GroupDocs.Merger Cloud SDK for Java, you can easily split PDF documents with just a few lines of code. PDF splitting is a common requirement in Java applications for extracting specific pages or sections from a PDF document. In this article, we will explore how to split PDF into multiple files in Java using REST API.
The following topics shall be covered in this article:
- Java REST API to Split PDF Files and SDK Installation
- Split Large PDF into Multiple Files in Java using REST API
Java REST API to Split PDF Files and SDK Installation
GroupDocs.Merger Cloud SDK for Java is a Java-based powerful and user-friendly library that allows you to manipulate PDF documents programmatically. It provides a wide range of features that make it easy to split, merge, reorder, rotate, swap, and manipulate PDF pages and documents. The SDK is easy to use and can be integrated into a Java-based application to automate file manipulation tasks.
You can either download the API’s JAR file or install it using Maven by adding the following repository and dependency into your project’s pom.xml file:
Maven Repository:
<repository>
<id>groupdocs-artifact-repository</id>
<name>GroupDocs Artifact Repository</name>
<url>https://repository.groupdocs.cloud/repo</url>
</repository>
Maven Dependency:
<dependency>
<groupId>com.groupdocs</groupId>
<artifactId>groupdocs-merger-cloud</artifactId>
<version>23.2</version>
<scope>compile</scope>
</dependency>
Next, you need to sign up for a free trial account or purchase a subscription plan on the GroupDocs website to get your API key. Once you have the Client Id and Client Secret, add below code snippet to a Java-based application:
Split Large PDF into Multiple Files in Java using REST API
For extracting specific pages or sections from a PDF document using GroupDocs.Merger Cloud SDK for Java, you will need to follow these simple steps:
- Upload the PDF files to the cloud
- Split PDF files into multiple documents in Java
- Download the PDF documents
Upload the Files
Firstly, upload the PDF files to the cloud using the code example given below:
As a result, the uploaded PDF file will be available in the files section of your dashboard on the cloud.
Separate PDF File into Multiple PDFs
Here are the steps and an example code snippet to split a PDF document into multiple files using GroupDocs.Merger Cloud SDK in your Java application:
- Firstly, import the required classes into your Java file.
- Secondly, create an instance of the DocumentApi class.
- Thirdly, create an instance of the FileInfo class.
- After that, set the input file path.
- Now, create an instance of the SplitOptions() class.
- Then, define split options setFileInfo and setPages collection in array format.
- Next, provide output file path and set the split options mode to INTERVALS.
- Now, create an instance of the SplitRequest() class and pass the SplitOptions parameter.
- Finally, split the PDF by calling the split() method of the DocumentApi and passing the SplitRequest parameter.
The following code snippet shows how to split PDF files online into multiple files in Java using REST API:
Download the File
The above code sample will save the split PDF file on the cloud. You can download it using the following code sample:
That’s it!
Free Online PDF Document Splitter
How to split PDF file into multiple files for free? Please try the online PDF splitter to split PDF into separate pages for free. No software installation required. This online document splitter is developed using the above-mentioned Groupdocs.Merger Cloud APIs.
Conclusion
PDF splitting is a crucial functionality in many Java applications, and GroupDocs.Merger Cloud SDK for Java provides a simple and efficient solution for document splitting in Java. The following is what you have learned in this article:
- how to split one PDF into multiple PDF files on the cloud using Java;
- programmatically upload and download the files in Java on the cloud;
- and split PDF files for free using an online PDF splitter.
Additionally, we also provide an API Reference section that lets you visualize and communicate with our APIs directly through the browser. Java SDK’s complete source code is freely available on Github. Please check the GroupDocs.Merger Cloud SDK for Java Examples here.
Moreover, we suggest you follow our Getting Started guide for detailed steps and API usage.
Finally, we keep writing new blog posts on different document operations using REST API. So, please get in touch for the regular updates.
Ask a question
If you have any questions about the PDF Document Splitter API, please feel free to ask us on the Free Support Forum.
FAQs
How do I split one PDF into multiple PDFs in Java?
You can split a PDF document into multiple files programmatically in Java using GroupDocs.Merger Cloud SDK for Java by calling the SplitRequest and Split document method with appropriate parameters such as the source file path, output file path, and split options like page numbers, page ranges etc.
Can I split a PDF document into multiple files with different page ranges?
Yes, you can split a PDF document into multiple files with different page ranges in Java using GroupDocs.Merger Cloud SDK for Java. You can specify multiple page ranges as split options in the SDK.
Can I split a PDF document into separate files based on specific page numbers?
Yes, you can split a PDF document into separate files in Java using GroupDocs.Merger Cloud SDK for Java by specifying the desired page numbers as split options.
See Also
Here are some related articles that you may find helpful:
- Combine and Merge PDF Files into One in Java using REST API
- How to Merge Word Documents (DOC, DOCX) in Java
- How to Split PowerPoint PPT or PPTX Slides in Python
- Extract Specific Pages from PDF using Python
- Extract Pages From Word Documents using Rest API
- Merge PowerPoint PPT/PPTX Files Online using REST API
- How to Change Page Orientation in Word Document using Ruby
- How to Split Word Document into Separate Files using Node.js
- Extract Pages from PDF in Java - Separate PDF Pages Online