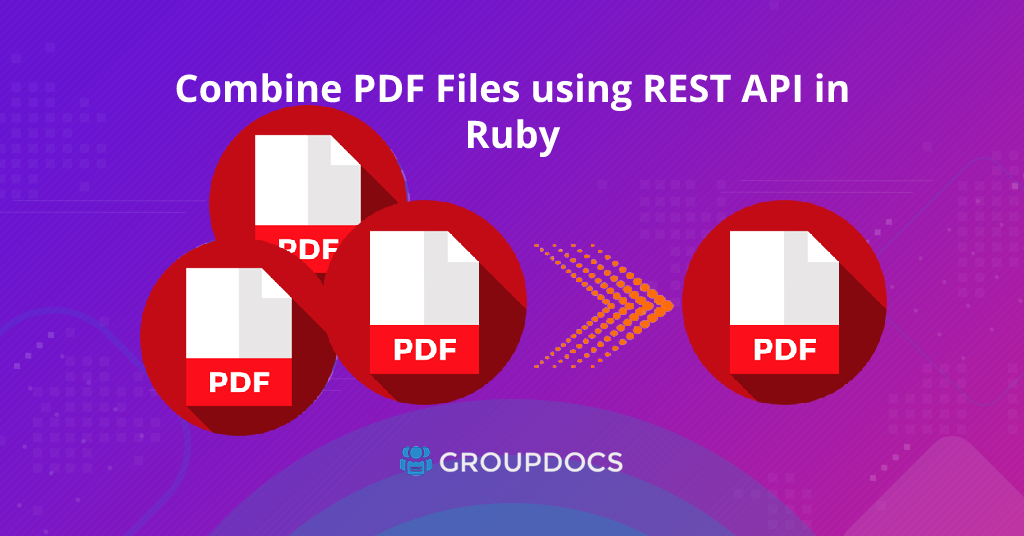
How to Merge and Combine PDF Files using REST API in Ruby
You can combine PDF documents into a single PDF file programmatically on the cloud using REST API. It can be useful in sharing or printing multiple documents combined in a single file instead of processing all files one by one. As a Ruby developer, you can merge two or more PDF files into a single file in your Ruby applications. In this article, you will learn how to merge and combine PDF files using REST API in Ruby.
The following topics shall be covered in this article:
- PDF Merger REST API and Ruby SDK
- Combine Multiple PDF Files using REST API using Ruby
- Merge Specific Pages of Multiple PDF Files using Ruby
- Online PDF Merger for Free
PDF Merger REST API and Ruby SDK
For merging two or more pdf files, I will be using the Ruby SDK of GroupDocs.Merger Cloud API. It allows you to combine two or more documents into a single document, or split up one source document into multiple resultant documents. It also enables you to shift, delete, exchange, rotate or change the page orientation either as portrait or landscape for the whole or preferred range of pages. The SDK supports merging and splitting of all popular document formats such as Word, Excel, PowerPoint, Visio, OneNote, HTML, etc.
You can install GroupDocs.Merger Cloud to your Ruby application using the following command in the console:
gem install groupdocs_merger_cloud
Please get your Client ID and Secret from the dashboard before following the mentioned steps. Once you have your ID and secret, add in the code as shown below:
Combine Multiple PDF Files using REST API in Ruby
You can combine two or more PDF files programmatically on the cloud by following the simple steps mentioned below. You can upload the PDF documents to the cloud and as a result, uploaded PDF files will be available in the files section of your dashboard on the cloud. You can easily merge multiple PDF documents into a single file programmatically by following the steps mentioned below:
- Create an instance of the DocumentApi
- Create an instance of the JoinItem
- Set the input file path for first JoinItem in the FileInfo
- Create new instance of the JoinItem for the second document
- Provide the input file path for second JoinItem in the FileInfo
- Add more JoinItems to merge more PDF files
- Create an instance of the JoinOptions
- Add a comma separated list of created join items
- Set the output file path on the cloud
- Create an instance of the JoinRequest with JoinOptions
- Get results by calling the join() method of the DocumentAPI with JoinRequest
The following code snippet shows how to merge multiple PDF files using a REST API in Ruby.
The above code sample will save the merged PDF files on the cloud.
Merge Specific Pages of Multiple PDF Files using Ruby
You can easily combine specific pages from multiple PDF files into a single file programmatically by following the steps mentioned below:
- Create an instance of the DocumentApi
- Create an instance of the JoinItem
- Set the input file path for first JoinItem in the FileInfo
- Define a list of page numbers to be merged
- Create another instance of the JoinItem
- Set the input file path for second JoinItem in the FileInfo
- Define start page number and end page number
- Define the page range mode as OddPages
- Create an instance of the JoinOptions
- Add a comma separated list of created join items
- Set the output file path on the cloud
- Create an instance of the JoinRequest with JoinOptions
- Finally, merge documents by calling the join() method of the DocumentAPI with JoinRequest
The following code snippet shows how to merge specific pages from multiple PDF files using a REST API in Ruby.
Online PDF Merger for Free
Please try the following free online PDF merging tool, which is developed using the above API. You can combine PDF online from any device using our PDF Merger tool.
Summing up
In this blog post, we have learned how to merge multiple PDF files on the cloud. We have also learned how to combine specific pages of multiple PDF documents into one file using Ruby. The PDF merger REST API also provides .NET, Java, PHP, Python, Android, and Node.js SDKs as its document merger family members for the Cloud API. You can learn more about GroupDocs.Merge Cloud API using the documentation. We also provide an API Reference section that lets you visualize and interact with our APIs directly through the browser.
Ask a question
If you have any questions about how to combine multiple PDF documents, please feel free to ask in Free Support Forum and it will be answered within a few hours.