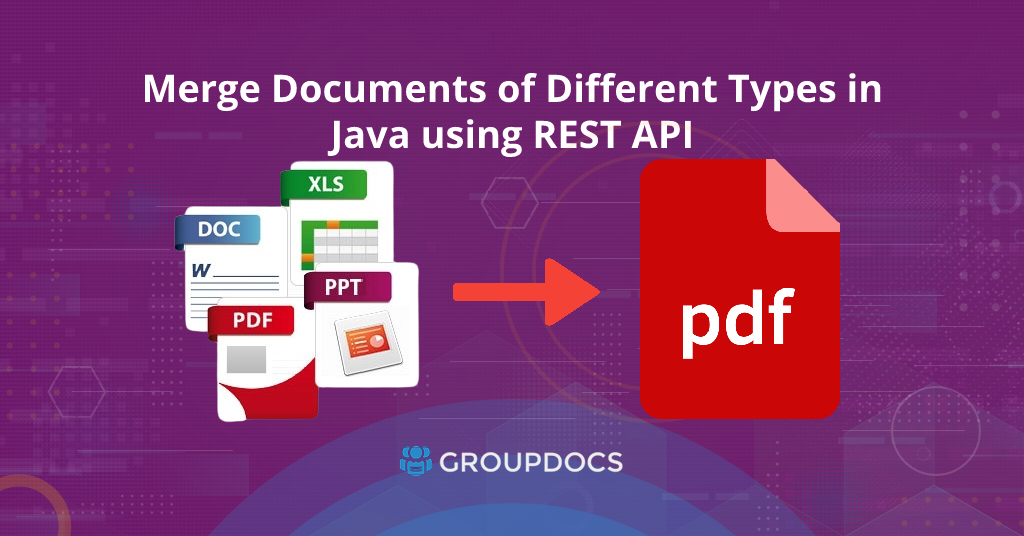
Merge Documents of Different Types in Java using REST API.
In today’s digital world, managing and manipulating various document types is a common requirement for many applications. Document merging is the process of combining multiple documents into a single document, thereby creating a consolidated file that includes the content of all the merged files. Fortunately, GroupDocs.Merger Cloud SDK for Java allows developers to merge various file formats such as PDF, Word, Excel, PowerPoint, and more, making it easier to handle and share information. In this article, we will explore how to merge multiple files into one document in Java using GroupDocs.Merger Cloud SDK for Java.
The following topics shall be covered in this article:
- Java REST API to Merge Multiple Documents and SDK Installation
- Merge Multiple File Types into One PDF in Java using REST API
Java REST API to Merge Multiple Documents and SDK Installation
GroupDocs.Merger Cloud SDK for Java is a powerful and feature-rich cloud-based tool that allows users to merge multiple file types into one document effortlessly. It allows you to merge, extract, swap, split, rearrange, delete, and change the orientation of the pages. Additionally, developers can define the merge order, specify page ranges, exclude specific pages, rearrange pages as needed, and more. The SDK is easy to use and offers seamless integration with Java applications.
You can either download the API’s JAR file or install it using Maven by adding the following repository and dependency into your project’s pom.xml file:
Maven Repository:
<repository>
<id>groupdocs-artifact-repository</id>
<name>GroupDocs Artifact Repository</name>
<url>https://repository.groupdocs.cloud/repo</url>
</repository>
Maven Dependency:
<dependency>
<groupId>com.groupdocs</groupId>
<artifactId>groupdocs-merger-cloud</artifactId>
<version>23.2</version>
<scope>compile</scope>
</dependency>
Now, sign up for a free trial account or purchase a subscription plan on the GroupDocs website and get your API key. Once you have the Client Id and Client Secret, add below code snippet to a Java-based application:
Merge Multiple File Types into One PDF in Java using REST API
Merging multiple documents in Java using GroupDocs.Merger Cloud SDK is straightforward. Follow the steps below:
Upload the Files
Firstly, upload the files to the cloud using the code example given below:
As a result, the uploaded files will be available in the files section of your dashboard on the cloud.
Merge Multiple Documents Into One PDF in Java
Here are steps and a sample code snippet demonstrating how to merge multiple files into one document using GroupDocs.Merger Cloud SDK for Java:
- Firstly, import the required classes into your Java file.
- Secondly, create an instance of the DocumentApi class.
- Thirdly, create an instance of the FileInfo class.
- Next, call the setFilePath() method and pass the input file path as a parameter.
- Now provide the password of the PDF document.
- Then, create an instance of the JoinItem class.
- Now, call the setFileInfo() method and pass the fileInfo1 parameter.
- Next, create a second instance of the FileInfo and JoinItem classes.
- Next, set the input file path and fileInfo2 parameters.
- Add more JoinItems for merging more than two documents.
- After that, create an instance of the JoinOptions() class.
- Then, add a comma-separated list of created join items.
- Next, set the resultant file path.
- Now, create an instance of the JoinRequest() class and pass the JoinOptions parameter.
- Finally, merge all documents in one PDF file by calling the join() method of the DocumentApi and passing the JoinRequest parameter.
The following code snippet shows how to merge multiple files into one PDF document in Java using REST API:
Download the File
The above code sample will save the merged document on the cloud. You can download it using the following code sample:
Free Online Documents Merger
How to merge documents online for free? Please try the online document merger to join multiple files into one document for free. This online document merger is developed using the above-mentioned Groupdocs.Merger Cloud APIs.
Conclusion
In conclusion, GroupDocs.Merger Cloud SDK for Java is a powerful tool that simplifies the process of merging documents of different types in Java. The following is what you have learned in this article:
- how to combine multiple files into one PDF on the cloud using Java;
- programmatically upload and download the merged files in Java;
- and merge different files for free using an online document merger.
Moreover, we also provide an API Reference section that lets you visualize and communicate with our APIs directly through the browser. Java SDK’s complete source code is freely available on Github. Please check the GroupDocs.Merger Cloud SDK for Java Examples here.
Additionally, we suggest you follow our Getting Started guide for detailed steps and API usage.
Finally, we keep writing new blog posts on different document operations using REST API. So, please get in touch for the latest updates.
Ask a question
If you have any questions about the Online Document Merger API, please feel free to ask us on the Free Support Forum.
FAQs
Can I merge documents of different formats using GroupDocs.Merger Cloud SDK for Java?
Yes, GroupDocs.Merger Cloud SDK for Java supports merging documents of various formats, including PDF, Word, Excel, PowerPoint, and many more.
Can I merge password-protected documents using GroupDocs.Merger Cloud SDK for Java?
Yes, GroupDocs.Merger Cloud SDK for Java provides an option to merge password-protected documents, enhancing its security.
Can I specify the order of the documents to be merged using GroupDocs.Merger Cloud SDK for Java?
Yes, GroupDocs.Merger Cloud SDK for Java allows developers to specify the order in the documents to be merged, ensuring flexibility and control over the documents merging.
See Also
For further information on related topics, we suggest taking a look at the following articles:
- How to Rotate PDF Pages in Java using Rest API
- How to Split PowerPoint PPT or PPTX Slides in Python
- Extract Pages From Word Documents using Rest API
- Merge and Combine PDF Files using REST API in Ruby
- Java Document Splitting API - Split PDF into Multiple Files in Java
- Combine and Merge PDF Files into One in Java using REST API
- Extract Pages from PDF in Java - Separate PDF Pages Online
- Merge Multiple JPG Files into One in Java - Merge JPG to JPG