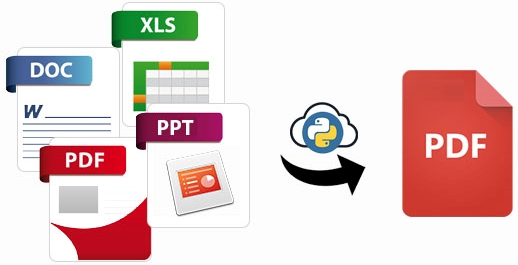
The merging of different documents of the same or different types allows gathering the scattered data or information into one single file. We can easily merge multiple documents of different file types into one file on the cloud. In this article, we will learn how to merge documents of different file types into PDF using a REST API in Python.
The following topics shall be covered in this article:
- File Merger REST API and Python SDK
- Merge Multiple File Types using REST API in Python
- How to Merge PDF and Excel into PDF
- How to Merge PDF and PowerPoint into PDF
- Combine Specific Pages of Different File Types in Python
File Merger REST API and Python SDK
For merging multiple files, we will be using the Python SDK of GroupDocs.Merger Cloud API. It enables us to combine, split, remove and rearrange a single page or a collection of pages from supported document formats of Word, Excel, PowerPoint, Visio drawings, PDF, and HTML. Please install it using the following command in the console:
pip install groupdocs-merger-cloud
Please get your Client ID and Secret from the dashboard before following the mentioned steps. Once you have your ID and secret, add in the code as shown below:
Merge Multiple File Types using REST API in Python
We can combine documents of multiple files types programmatically on the cloud by following the steps given below:
Upload the Files
Firstly, we will upload the files to the cloud using the code example given below:
As a result, the uploaded files will be available in the files section of your dashboard on the cloud.
Merge Documents of Different File Types in Python
Now, we can easily merge the uploaded files of different types into a single file by following the steps given below:
- Firstly, create an instance of the DocumentApi.
- Next, provide the input file path for first JoinItem.
- Then, provide the input file path for second JoinItem.
- Optionally, repeat the above steps to add more files.
- After that, define the JoinOptions and set the path of the output file.
- Finally, call the join() method and save the merged document.
The following code sample shows how to merge different file types using a REST API in Python.
Download the Merged File
Finally, the above code sample will save the merged PDF file on the cloud. It can be downloaded using the following code sample:
How to Merge PDF and Excel into PDF
We can merge PDF and Excel files into a PDF by following the steps mentioned earlier. However, we just need to provide PDF and Excel document paths as the first and second JoinItems. The following code sample shows how to merge a PDF document and Excel sheet into a PDF file using a REST API in Python.
How to Merge PDF and PowerPoint into PDF
We can also merge PDF documents and PowerPoint presentations into PDF by following the steps mentioned earlier. However, we just need to provide PDF and PowerPoint document paths as the first and second JoinItems. The following code sample shows how to merge a PDF document and a PowerPoint presentation into a PDF file using a REST API in Python.
Combine Specific Pages of Different Files Types in Python
We can merge selected pages from documents of different types into a single file by following the steps given below:
- Firstly, create an instance of the DocumentApi.
- Next, provide the input file path for first JoinItem.
- Then, provide specific page numbers to merge.
- Next, provide the input file path for second JoinItem.
- Then, define the pages range to merge with start page number and end page number.
- After that, define the JoinOptions and set the path of the output file.
- Finally, call the join() method and save the merged document.
The following code sample shows how to merge specific pages of different file types using a REST API in Python.
Try Online
Please try the following free online merging tool, which is developed using the above API. https://products.groupdocs.app/merger/
Conclusion
In this article, we have learned:
- how to merge documents of multiple file types in Python;
- how to combine specific pages from documents of different file types in Python;
- upload multiple files to the cloud;
- how to download merged PDF from the cloud.
Besides, you can learn more about GroupDocs.Merge Cloud API using the documentation. We also provide an API Reference section that lets you visualize and interact with our APIs directly through the browser. In case of any ambiguity, please feel free to contact us on the fo.