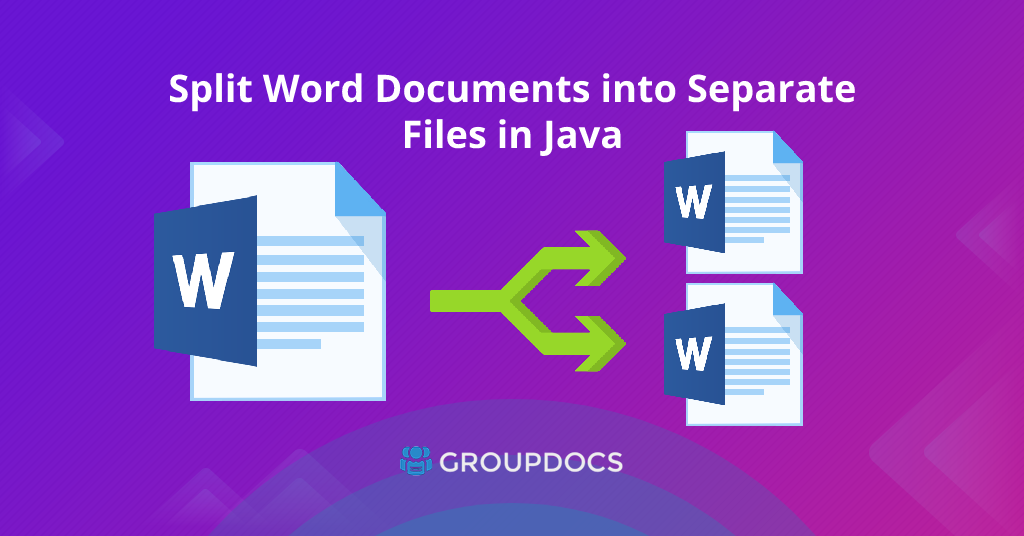
Split Word Documents into Separate Files in Java.
Are you looking for a reliable and easy way to split your Word documents in Java? GroupDocs.Merger Cloud SDK for Java provides a solution for splitting Word documents into multiple files quickly and easily. Splitting a Word document into multiple files can be useful for various reasons, such as splitting a large document into smaller documents, extracting specific pages or sections, or creating individual files for each section of a book. In this tutorial, we will explore how to split Word documents into separate files in Java using REST API.
The following topics shall be covered in this article:
- Java Word Documents Splitter REST API and SDK Installation
- Split Word Documents into Multiple Pages Documents using Java
- How to Split Word Documents into Separate Files Online in Java
- Split Word File Online to Single Pages by Range and Filter in Java
Java Word Documents Splitter REST API and SDK Installation
GroupDocs.Merger Cloud SDK for Java is a cloud-based powerful API that allows developers to merge, split, reorder, and remove pages from documents in various formats, including Word, Excel, PowerPoint, HTML, PDF, and many more. The SDK is easy to use and can be integrated into a Java-based application to automate file manipulation tasks.
You can either download the API’s JAR file or install it using Maven by adding the following repository and dependency into your project’s pom.xml file:
Maven Repository:
<repository>
<id>groupdocs-artifact-repository</id>
<name>GroupDocs Artifact Repository</name>
<url>https://repository.groupdocs.cloud/repo</url>
</repository>
Maven Dependency:
<dependency>
<groupId>com.groupdocs</groupId>
<artifactId>groupdocs-merger-cloud</artifactId>
<version>23.2</version>
<scope>compile</scope>
</dependency>
Before we can start using the GroupDocs.Merger Cloud SDK for Java, we need to sign up for a free trial account or purchase a subscription plan on the GroupDocs website to get your API key. Once you have the Client Id and Client Secret, add below code snippet to a Java-based application:
Split Word Documents into Multiple Pages Documents using Java
To split Word (DOC, DOCX) documents using GroupDocs.Merger Cloud SDK for Java, you will need to follow below simple steps:
- Upload the Word files to the cloud
- Split Word files into multiple documents in Java
- Download the Word documents
Upload the Files
Firstly, upload the Word files to the cloud using the code example given below:
As a result, the uploaded DOCX file will be available in the files section of your dashboard on the cloud.
Split Word Files into Multiple Documents in Java
Follow below step-by-step guide and an example code snippet to split Word documents into multipage documents in Java using GroupDocs.Merger Cloud SDK:
- Firstly, import the required classes into your Java file.
- Secondly, create an instance of the DocumentApi class.
- Thirdly, create an instance of the FileInfo class.
- After that, set the input file path.
- Now, create an instance of the SplitOptions() class.
- Then, define split options setFileInfo and setPages collection in array format.
- Next, provide output file path and set the split options mode to INTERVALS.
- Now, create an instance of the SplitRequest() class and pass the SplitOptions parameter.
- Finally, split DOCX files by calling the split() method of the DocumentApi and passing the SplitRequest parameter.
The following code snippet shows how to split Word files into multipage documents in Java using REST API:
Download the File
The above code sample will save the split Word file on the cloud. You can download it using the following code sample:
That’s it! Now you know how to split DOC or DOCX into multiple files using the GroupDocs.Merger Cloud SDK for Java.
How to Split Word Documents into Separate Files Online in Java
This section is about how to split Word documents into one-page documents in Java using GroupDocs.Merger Cloud SDK:
- Firstly, import the required classes into your Java file.
- Secondly, create an instance of the DocumentApi class.
- Thirdly, create an instance of the FileInfo class.
- After that, set the input file path.
- Now, create an instance of the SplitOptions() class.
- Then, define split options setFileInfo and setPages collection in array format.
- Next, provide output file path and set the split options mode to PAGES.
- Now, create an instance of the SplitRequest() class and pass the SplitOptions parameter.
- Finally, split DOCX files by calling the split() method of the DocumentApi and passing the SplitRequest parameter.
The following code snippet shows how to split Word documents into separate files in Java using REST API:
Split Word File Online to Single Pages by Range and Filter in Java
In this section we will cover step-by-step guide and an example code snippet to split Word documents into single page documents using Java by applying range and filter:
- Firstly, import the required classes into your Java file.
- Secondly, create an instance of the DocumentApi class.
- Thirdly, create an instance of the FileInfo class.
- After that, set the input file path.
- Now, create an instance of the SplitOptions() class.
- Then, define split options setFileInfo and output file path.
- Set values for setStartPageNumber and setEndPageNumber.
- Next, set page options setRangeMode to ODDPAGES and set the split options mode to PAGES.
- Now, create an instance of the SplitRequest() class and pass the SplitOptions parameter.
- Lastly, split DOCX files by calling the split() method of the DocumentApi and passing the SplitRequest parameter.
The following code snippet shows how to split DOCX file online to single pages by applying range and filter using Java:
Free Online Word Document Splitter
How to split Word online into multiple files for free? Please try the online Word DOCX splitter to separate Word documents into multiple files for free. This online document splitter is developed using the above-mentioned Groupdocs.Merger Cloud APIs.
Conclusion
GroupDocs.Merger Cloud SDK for Java provides an easy and reliable way to split Word documents in Java. The following is what you have learned in this article:
- how to split Word document into multiple Word documents on the cloud using Java;
- programmatically upload and download the documents using Java on the cloud;
- Split Word files into separate files online by page numbers using Java;
- Split Word DOCX into single page documents in Java by applying range and filter;
- and split Word files online for free using a free Word splitter online.
Additionally, we also provide an API Reference section that lets you visualize and communicate with our APIs directly through the browser. Java SDK’s complete source code is freely available on Github. Please check the GroupDocs.Merger Cloud SDK for Java Examples here.
Moreover, we suggest you follow our Getting Started guide for detailed steps and API usage.
Finally, we keep writing new blog posts on different document operations using REST API. So, please get in touch for the regular updates.
Ask a question
If you have any questions about the Word Splitter API, please feel free to ask us on the Free Support Forum.
FAQs
Is GroupDocs.Merger Cloud SDK for Java a paid API?
Yes, GroupDocs.Merger Cloud SDK for Java is a paid API, but it offers a free trial version that allows you to test its features before making a purchase.
Is it possible to split Word DOCX into multiple files in Java?
Yes, you can split a Word document into multiple files programmatically in Java using GroupDocs.Merger Cloud SDK for Java.
Can I split other file formats using GroupDocs.Merger Cloud SDK for Java?
Yes, you can split PDF, Excel, PowerPoint, and other file formats using GroupDocs.Merger Cloud SDK for Java.
See Also
Here are some related articles that you may find helpful:
- How to Merge Word Documents (DOC, DOCX) in Java
- How to Split PowerPoint PPT or PPTX Slides in Python
- Extract Specific Pages from PDF using Python
- Extract Pages From Word Documents using Rest API
- Merge PowerPoint PPT/PPTX Files Online using REST API
- How to Change Page Orientation in Word Document using Ruby
- How to Split Word Document into Separate Files using Node.js