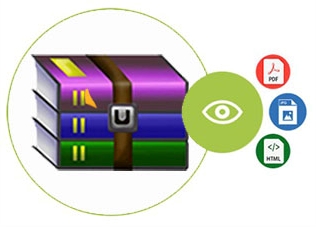
We can store one or more files or folders compressed in a ZIP file to act as a single file. The ZIP archive saves storage space and increases the performance of computers. It also allows us to transfer our files and folders in a ZIP archive from one location to another effectively. In this article, we will learn how to view the content of ZIP files using a REST API in Python.
The following topics shall be covered in this article:
- ZIP File Viewer REST API and Python SDK
- View ZIP Files in HTML using REST API in Python
- View Specific Folder from ZIP Archives in HTML
- Render Content of ZIP Files in PDF
- Render ZIP Archives to JPG
- Get a List of Files and Folders from ZIP Archives
ZIP File Viewer REST API and Python SDK
For rendering ZIP archives, we will be using the Python SDK of GroupDocs.Viewer Cloud API. It enables us to programmatically render all sorts of popular document formats. Please install it using the following command in the console:
pip install groupdocs-viewer-cloud
Please get your Client ID and Secret from the dashboard before following the mentioned steps. Once you have your ID and secret, add in the code as shown below:
View ZIP Files in HTML using REST API in Python
We can render ZIP archives in HTML by following the simple steps given below:
- Upload the ZIP file to the cloud
- Render ZIP to HTML
- Download the rendered HTML file
Upload the ZIP File
Firstly, we will upload the ZIP file to the cloud using the code example given below:
As a result, the uploaded file will be available in the files section of the dashboard on the cloud.
Render ZIP to HTML in Python
Now, we will view the content of the uploaded ZIP archive in the browser by following the steps given below:
- Firstly, create an instance of the ViewAPI.
- Next, define the view_options and provide the uploaded ZIP file path.
- Then, set the view_format as “HTML”.
- Optionally, set render_to_single_page to True.
- After that, create CreateViewRequest with view_options as argument.
- Finally, render ZIP to HTML using the **create_view()** method.
The following code sample shows how to render the ZIP file in HTML using a REST API in Python.
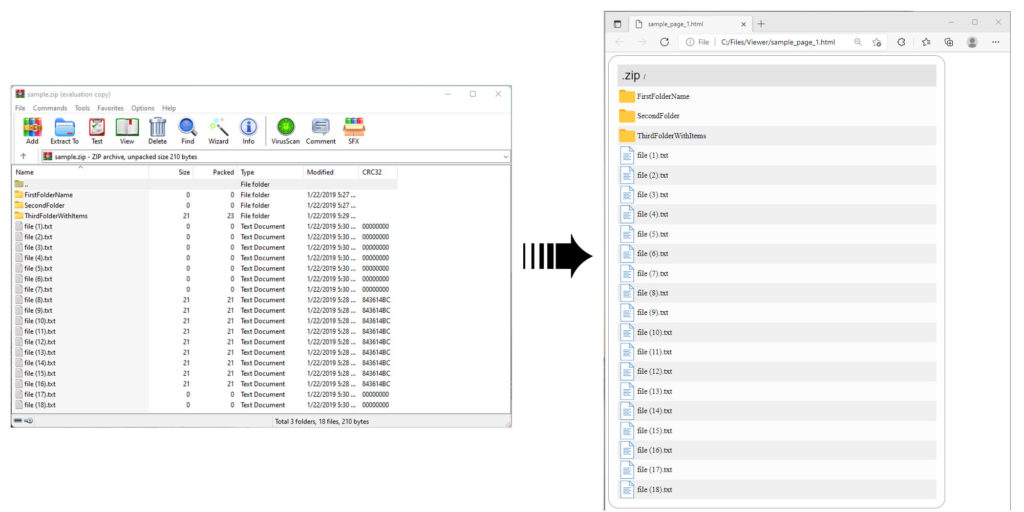
View ZIP Files in HTML using REST API in Python.
Download the Rendered File
The above code sample will save the rendered HTML file on the cloud. It can be downloaded using the following code sample:
View Specific Folder from ZIP Archives in HTML
We can also view only a specific folder from the ZIP file in the browser by following the steps given below:
- Firstly, create an instance of the ViewAPI.
- Next, define view_options and provide the uploaded ZIP file path.
- Then, set the view_format as “HTML”.
- Next, define ArchiveOptions and provide the folder name to render.
- After that, create CreateViewRequest with view_options as argument.
- Finally, render a specific folder from ZIP to HTML using the create_view() method.
The following code sample shows how to render a specific folder from the ZIP file in HTML using Python.
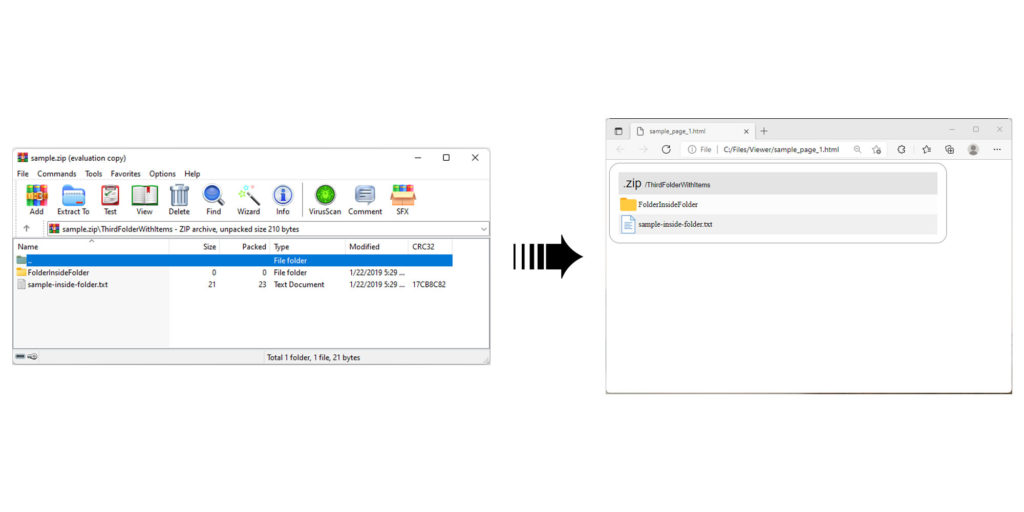
View Specific Folder from ZIP Archives in HTML.
Render Content of ZIP Files in PDF
We can render the content of a ZIP file in a PDF document by following the simple steps given below:
- Firstly, create an instance of the ViewAPI.
- Next, define view_options and provide the uploaded ZIP file path.
- Then, set the view_format as “PDF”.
- After that, create CreateViewRequest with view_options as argument.
- Finally, render content from ZIP to PDF using the create_view() method.
The following code sample shows how to render the content of a ZIP file in PDF using a REST API in Python.
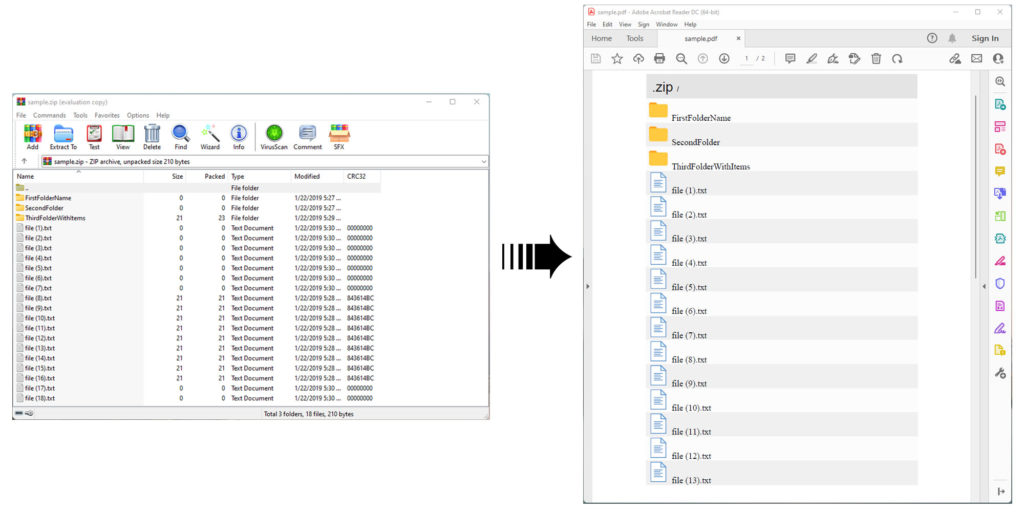
Render Content of ZIP Files in PDF.
Render ZIP Archives to JPG
We can also render the content of a ZIP file as a JPG image by following the steps given below:
- Firstly, create an instance of the ViewAPI.
- Next, define view_options and provide the uploaded ZIP file path.
- Then, set the view_format as “JPG”.
- After that, create CreateViewRequest with view_options as argument.
- Finally, render ZIP to JPG using the create_view() method.
The following code sample shows how to render the ZIP file in a JPG image using a REST API in Python.
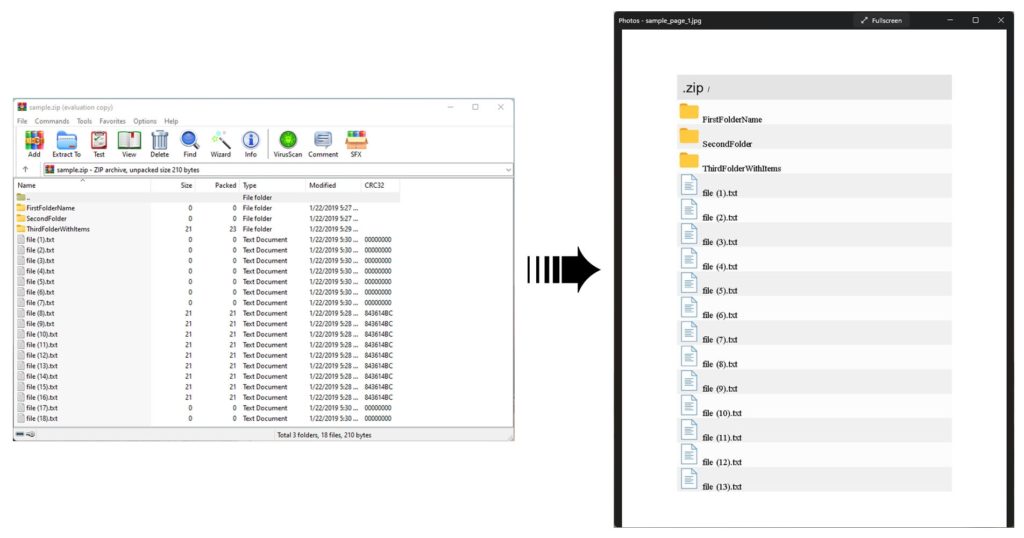
Render ZIP Archives to JPG.
Get a List of Files and Folders from ZIP Archives
We can get a list of all the files and folders from the ZIP archive by following the steps given below:
- Firstly, create an instance of the InfoAPI.
- Next, define view_options and provide the uploaded ZIP file path.
- After that, create GetInfoRequest with view_options as argument.
- Finally, list the content of a ZIP file using the get_info() method.
The following code sample shows how to get a list of files and folders from the ZIP file in Python.
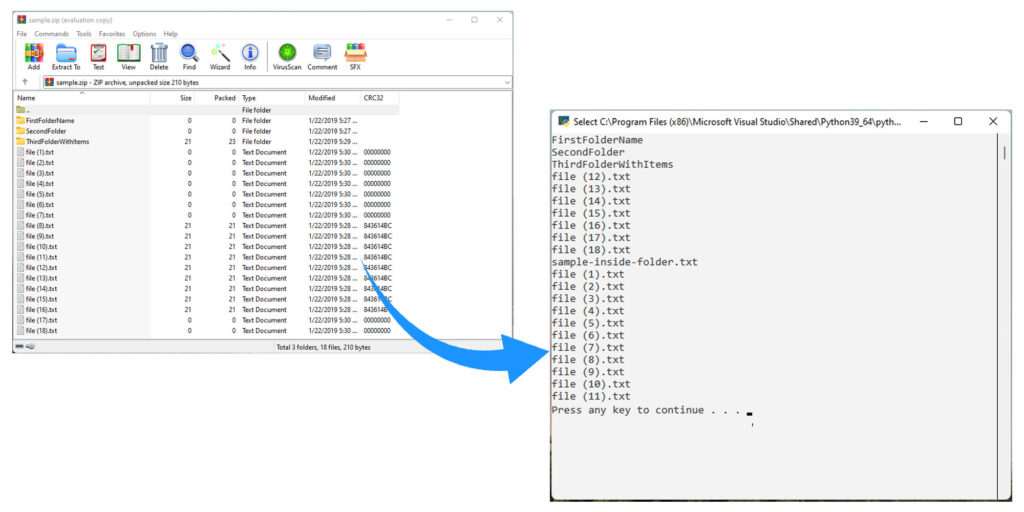
Get a List of Files and Folders from ZIP Archives.
Try Online
Please try the following free online ZIP rendering tool, which is developed using the above API. https://products.groupdocs.app/viewer/zip
Conclusion
In this article, we have learned how to:
- render ZIP archive or a specific folder from ZIP to HTML in Python;
- view the content of a ZIP file in PDF;
- render ZIP archives to JPG;
- list the files and folders of a ZIP archive;
- programmatically upload the ZIP file to the cloud;
- download the rendered HTML file from the cloud.
Besides, you can learn more about GroupDocs.Viewer Cloud API using the documentation. We also provide an API Reference section that lets you visualize and interact with our APIs directly through the browser. In case of any ambiguity, please feel free to contact us on the forum.